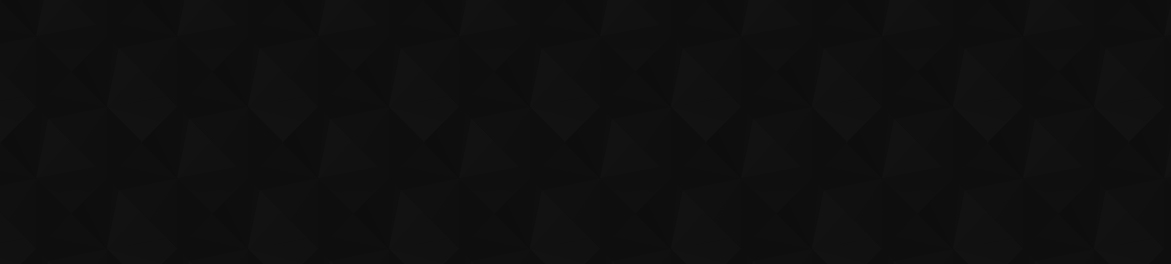
- Видео 137
- Просмотров 33 214
metameeee
США
Добавлен 4 сен 2023
I mostly stream on Twitch and upload some VODs here. I'm a backend developer with 10+ years of experience. I've written a few different languages over the years (Java(Script), TypeScript, Clojure, Haskell) but mostly stream Rust and Functional Programming nonsense. Come check me out live or just subscribe here and watch my vids/vods/vads.
Implementing a Ring Buffer in Rust 🦀! - Rust DSA v2 (part 7)
Implementing Ring Buffer, Circle Buffer, Array Buffer, etc. in Rust! This is one of my favorite data structures because it uses an array (or at least an array-like contiguous memory allocation) in a clever way to provide constant time access to both sides of a data set. Thanks for watching!
My article on RingBuffer: metame.substack.com/p/rust-dsa-ring-buffer
Github: github.com/metame/rust-dsa-live/blob/main/src/ring_buffer.rs
Previous episodes:
Linear & Binary Search: ruclips.net/video/oV-_pnOApo8/видео.html
Singly Linked List: ruclips.net/video/MPm04xkiPbA/видео.html
Doubly Linked List: ruclips.net/video/hUbslbgjXt4/видео.html
RawVec: ruclips.net/video/lO7VKwCydnA/видео.html
ArrayList: ruclips.n...
My article on RingBuffer: metame.substack.com/p/rust-dsa-ring-buffer
Github: github.com/metame/rust-dsa-live/blob/main/src/ring_buffer.rs
Previous episodes:
Linear & Binary Search: ruclips.net/video/oV-_pnOApo8/видео.html
Singly Linked List: ruclips.net/video/MPm04xkiPbA/видео.html
Doubly Linked List: ruclips.net/video/hUbslbgjXt4/видео.html
RawVec: ruclips.net/video/lO7VKwCydnA/видео.html
ArrayList: ruclips.n...
Просмотров: 346
Видео
Writing an article on Ring Buffer in Rust 🦀! - Rust DSA Blog (part 7)
Просмотров 10214 дней назад
Writing about Ring Buffer, Circle Buffer, Array Buffer, etc. This is one of my favorite data structures because it uses an array (or at least an array-like contiguous memory allocation) in a clever way to provide constant time access to both sides of a data set. Implementation video coming! This post is already live with the code: metame.substack.com/p/rust-dsa-ring-buffer Follow along as we im...
How do BigNum Libraries Work??
Просмотров 42114 дней назад
I thought this was a nice article to read, not too long, not too short. I didn't really think about storing bignums as a string before, but it should work fine depending on how strings are represented in the programming language you've chosen. Hope you enjoy! Article link: austinhenley.com/blog/bignum1.html Watch live at www.twitch.tv/metameeee metameeee
Implementing & Benchmarking Stacks & Queues in Rust 🦀! - Rust DSA v2 (part 6)
Просмотров 53821 день назад
We use the Linked Lists and Array List we wrote to implement FiFo queues and LiFo queues (aka stacks)! The implementation is *very* simple and the benchmarks have a few surprising results! We use criterion for the benchmarks and it's pretty simple to use and get started with. Thanks for watching! My article on Stacks & Queues: metame.substack.com/p/rust-dsa-queues-and-stacks Github Stack: githu...
Writing an article on Queues & Stacks in Rust 🦀! - Rust DSA Blog (part 6)
Просмотров 6321 день назад
Writing about FiFo queues and LiFo queues (aka stacks). We go over some of the differences in performance and what data structures lend themselves to efficiency for these two very practical data structures. Implementation video coming! This post is already live with the code: metame.substack.com/p/rust-dsa-queues-and-stacks Follow along as we implement data structures and algorithms in Rust! Wa...
Fixing DOUBLE FREE & MEM LEAK in ArrayList in UNSAFE Rust with Miri 🦀! - Rust DSA v2 (part 5a)
Просмотров 34928 дней назад
Let's tackle some UB we caused with the Iterator implementation and make sure we don't have any memory leaks when using a complex type like String. This is a fun one for learning miri and seeing it in action as it guides me to fix (hopefully) all of the memory issues I've caused. My article on ArrayList: metame.substack.com/p/rust-dsa-array-list Github: github.com/metame/rust-dsa-live/blob/main...
ArrayList in UNSAFE Rust 🦀! - Rust DSA v2 (part 5)
Просмотров 549Месяц назад
We implement an Array List in Rust! An Array List is just like a Vec (from Rust's stdlib). The basic implementation goes quickly so we also implement a consuming iterator, Index/IndexMut traits, and push_front (an inefficient way of adding an element). Note: The ArrayList on stream is a little different than the final implementation on the github due to a few UB and memory leak issues with the ...
Writing an article on ArrayList/Vec in Rust 🦀! - Rust DSA Blog (part 5)
Просмотров 34Месяц назад
We write about an Array List, aka Vec (in Rust), Dynamic Array, DynArray, among other names. Implementation video coming! This post is already live with the code: metame.substack.com/p/rust-dsa-array-list Follow along as we implement data structures and algorithms in Rust! Watch live at www.twitch.tv/metameeee metameeee
Rust on Easy Mode?! - Announcing June Lang (prerelease)
Просмотров 91Месяц назад
We talk about that (as yet unfinished) June lang, a safe systems language that uses grouped allocations in order to provide an easier development experience (as compared to Rust). Unfortunately, before I could post this video, this project was defunded and archived but I still think there's some interesting ideas here that are worth thinking about even if June will never see the light of day! A...
Growable Memory Buffer (RawVec) in UNSAFE Rust 🦀! - Rust DSA v2 (part 4)
Просмотров 95Месяц назад
We delve into how to allocate and deallocate memory using unsafe Rust in order to build a contiguous growable memory buffer that we can use for array-like data structures. My article on RawVec: metame.substack.com/p/rust-dsa-rawvec Github: github.com/metame/rust-dsa-live/blob/main/rawvec.rs Previous episodes: Linear & Binary Search: ruclips.net/video/oV-_pnOApo8/видео.html Singly Linked List: r...
Writing an article on RawVec in UNSAFE Rust 🦀! - Rust DSA Blog (part 4)
Просмотров 249Месяц назад
We write about how to manually allocate and reallocate contiguous memory blocks in Rust! Implementation video coming! This post is already live with the code: metame.substack.com/p/rust-dsa-rawvec Follow along as we implement data structures and algorithms in Rust! Watch live at www.twitch.tv/metameeee metameeee
Doubly Linked List in Rust 🦀! - Rust DSA v2 (part 3)
Просмотров 55Месяц назад
Never having significantly worked with Arc & Mutex, this was a bit of a challenge. It definitely required more syntax and less ergonomics than working with Rc/Refcell. Post-stream I found some terser ways of expressing the behavior which you can see on the github link below. Hope you enjoy this! My article on Doubly Linked List: metame.substack.com/p/rust-dsa-doubly-linked-list Github: github.c...
Writing an article on Doubly Linked List in Rust 🦀! - Rust DSA Blog (part 3)
Просмотров 127Месяц назад
The next challenge is the Doubly Linked List! We write about the challenges and solutions for managing multiple references in Rust when you need mutation. Implementation video coming! This post is already live with the code: metame.substack.com/p/rust-dsa-doubly-linked-list Follow along as we implement data structures and algorithms in Rust! Watch live at www.twitch.tv/metameeee met...
Singly Linked List in Rust 🦀! - Rust DSA v2 (part 2)
Просмотров 395Месяц назад
The famously perceived as difficult but completely whiteboard-able singly linked list in Rust! Box definitely takes out some of the complexity here and the basic linked list is a breeze! I do hit a couple annoying walls with not getting the right type of references in my split method but we get there! 00:00:00 - Reading Article 00:08:55 - Basic Linked List 00:57:30 - Split & Append My article o...
Writing an article on Singly Linked List in Rust 🦀! - Rust DSA Blog (part 2)
Просмотров 102Месяц назад
Linked lists are so simple but many would consider them difficult to implement in Rust! Hopefully, this article explains how simple they really are and the implementation will show how easy it is to make one in Rust! The implementation video is coming! This post is already live with the code: metame.substack.com/p/rust-dsa-linked-list Follow along as we implement data structures and algorithms ...
I Built Flakes for 21 OCaml Libraries in the OCaml Riot Stack! 🐪 (Part 13 - The Finale)
Просмотров 72Месяц назад
I Built Flakes for 21 OCaml Libraries in the OCaml Riot Stack! 🐪 (Part 13 - The Finale)
Building out Nix Flakes ❄️ for the OCaml Riot Stack! 🐪 (Part 12)
Просмотров 492 месяца назад
Building out Nix Flakes ❄️ for the OCaml Riot Stack! 🐪 (Part 12)
Linear & Binary Search in Rust 🦀! - Rust DSA v2 (part 1)
Просмотров 2092 месяца назад
Linear & Binary Search in Rust 🦀! - Rust DSA v2 (part 1)
Writing an article on Linear & Binary Search in Rust 🦀! - Rust DSA Blog (part 1)
Просмотров 582 месяца назад
Writing an article on Linear & Binary Search in Rust 🦀! - Rust DSA Blog (part 1)
Building out Nix Flakes ❄️ for the OCaml Riot Stack! 🐪 (Part 11)
Просмотров 2222 месяца назад
Building out Nix Flakes ❄️ for the OCaml Riot Stack! 🐪 (Part 11)
Building out Nix Flakes ❄️ for the OCaml Riot Stack! 🐪 (Part 10)
Просмотров 682 месяца назад
Building out Nix Flakes ❄️ for the OCaml Riot Stack! 🐪 (Part 10)
Planning a new Rust DSA series 🦀 (part 2)
Просмотров 742 месяца назад
Planning a new Rust DSA series 🦀 (part 2)
Building out Nix Flakes ❄️ for the OCaml Riot Stack! 🐪 (Part 9)
Просмотров 512 месяца назад
Building out Nix Flakes ❄️ for the OCaml Riot Stack! 🐪 (Part 9)
Invincible & Blazingly Fast?! Learning about Golem cloud - Rust WASM Component Platform 🦀
Просмотров 1132 месяца назад
Invincible & Blazingly Fast?! Learning about Golem cloud - Rust WASM Component Platform 🦀
Planning a new Rust DSA series 🦀 (and a little bit of algo stuff)
Просмотров 1532 месяца назад
Planning a new Rust DSA series 🦀 (and a little bit of algo stuff)
OCaml Bug Hunting for Serde & Riot & More Nix flakes 🐫 ❄️ (nix riot part 8)
Просмотров 763 месяца назад
OCaml Bug Hunting for Serde & Riot & More Nix flakes 🐫 ❄️ (nix riot part 8)
Speedrunning CS3110 - OCaml Programming Book 🐫 (Part 9) - Ch 5 Exercises
Просмотров 1993 месяца назад
Speedrunning CS3110 - OCaml Programming Book 🐫 (Part 9) - Ch 5 Exercises
FUNCTORS! Speedrunning CS3110 - OCaml Programming Book 🐫 (Part 8) - Ch 5.9 & Exercises
Просмотров 1213 месяца назад
FUNCTORS! Speedrunning CS3110 - OCaml Programming Book 🐫 (Part 8) - Ch 5.9 & Exercises
Building out Nix Flakes ❄️ for the OCaml Riot Stack! 🐪 (Part 7)
Просмотров 693 месяца назад
Building out Nix Flakes ❄️ for the OCaml Riot Stack! 🐪 (Part 7)
Speedrunning CS3110 - OCaml Programming Book 🐫 (Part 7) - Ch 5.4 - 5.8
Просмотров 1023 месяца назад
Speedrunning CS3110 - OCaml Programming Book 🐫 (Part 7) - Ch 5.4 - 5.8
Is Real World OCaml the other "most recommended" book? Thoroughly enjoying your videos; thanks for learning in public!
Glad you're enjoying them! Yes, Real World OCaml is the other one :)
noice
Yoo...thanks for this awesome playlist
dope you should fix the video order in the playlist tho
thanks for the heads up! should be fixed :)
I did a little bit of extra research after the video and it seems like storing a dynamically sized array of digits (0-9, not sure if I'd call that a "string" per se) is the way most languages implement it.
ya that makes sense! easy to leverage the way strings are represented in C in this case :)
zshhhhh.
sadly already deprecated :/
Ya, it's too bad but such is the OSS life.
good video!
Thanks emil
Great
This is good
no ocaml-bindgen sadge. It's an annoying one too. I can't get it to work on my voidlinux install because of a different name of the ncurses package it expects it cant find the system package so I've been dev-ing from a Kubuntu VM.
When are you planning to start?
The first video just went live!
You had me at Erlang! Nice video. Thanks for sharing / presenting. :)
Thanks for watching and the kind words!
lets go and was waiting for it!!
Lets goo 👏
Can you build projects using C++ too? Would really help me a lot
Not in my current plans, but who knows what the future holds
Which keyboard are you using? Looks a bit like the moonlander?
Ergodox EZ, precursor to moonlander :)
noice
Yo dude, are you okay ? Hope everything is okay. Thanks for the series
Do gleam/lustre, or rust/xilem. I'm struggling with UI'ing in my favorite languages... ^^'
Keep going bud
I wish to get to your level in Rust before 2025
Hey just want to ask will you continue this series ??
I'm planning on a new Rust DSA series that will be implementing several of these data structures again, aiming at a single 2-3 hour video per data structure then hopefully continue with some new data structures! Thanks for watching!
maybe i read too much of the linux coding style doc but holy moly are you using many nested statements.
Yes
linked list is the only way to get a dynamic list going in rust, if you dont want a vec!
We implement our own soon enough in the series :D
A heavy Indian accent or a big blonde beard on a white dude are the most reliable indicators of good technical content on RUclips.
Hi ! love your content! what s the font you re using ?
It's Andale Mono. Thanks for watching!
@@metameeeeOH i meant the one you have in your terminal and neovim !
Ah, yes that's JetBrainsMono :)@@mehdiboujid8761
What font is that? Looks good.
It's Andale Mono. Thanks for watching!
Cool vid, what font is that on your editor?
andale mono - thanks for watching!
Just Found your playlist, excited!
Definitely a playlist of me learning about Rust and Data Structures, so get ready for the skill issues :) Thanks for watching!
@@metameeee I would really appreciate if you could recommend me whether I should do DSA in Rust and if yes then please be kind enough to provide some other resources along with your lectures.
I followed along ThePrimeagen's course on DSA, which is free on Frontend Masters, just to learn/relearn the data structures, then I implemented them in Rust through grinding :D
@@metameeee Thanks a lot for your suggestion as well as quick reply. If you don't mind me asking, what are you doing nowadays?
hey sorry if I'm spamming your comments section, but I'm really glad I found your channel and these videos. I have been looking to write some web apps using Zig but found no reference how to do that. this video might be it!
Glad you're enjoying them! Lots of skill issues but also a lot of fun :)
oh woww you're doing Zig as well!! awesome stuff...liked and subbed!
hey I just found your channel and I got to say I love this series. I'm currently learning C, Rust and Zig as part of my low level learning. C should be easy for you since you write Rust. great video, more please!
What font are you using in vim?
my editor font is Andale Mono (it's technically emacs with evil-mode/vim motions but don't tell anyone 😉)
Keep going 👏
gcc on macos is aliased to clang by default???
Indeed... Apple clang to be precise 😀
I'm a metalhead but I'd rather have VODs without any music. Maybe it's just me but I find it very distracting when I'm trying to listen to someone talking.
Hey there, thanks for the feedback! I've been considering uploading a version without music as well. Maybe this is a push to do that 🙂
Have u tried Lean 4?
Haven't tried it but on a list for the future ;)
I'm gonna beat you in Rust someday.. I'll reach your level 🧐
Now the tables are turned.
It would be super helpful in all of these videos if you shared the timestamp for when you actually start. 00:00 Getting Started ?? Actual Start 12:50 Setting up repo 15:05 Series Intro 24:04 C
Hey, thanks for watching. The second timestamp is my "actual start" from my perspective. But maybe you mean just when I actually get back on camera and start talking? Usually before that is me just talking about random stuff with chat :D
@@metameeeeAh, thanks. I suppose I was looking for a place where you give an overview of what you're doing, what resources you're using and such. Is the idea that folx just follow along, or that there is a resource that we kinda just do in parallel. How do you intend these to be used?
@@Mugabo2023 definitely more just fun and exploration than anything else. But the github repo can be a nice reference to track progress (and eventually the README's will be improved) - github.com/metame/mtol
Where do you stream? Any way to support you, donate something or buy you a coffee?
Ah very kind. I stream on twitch.tv/metameeee. Hope to see you live sometime! I do in fact have a ko-fi.com/metameeee for just that reason. Thanks for watching.
Your alone on youtube with this detailed dsa rust content, you deserve so much more views. Thanks for teaching us
Thank you so much for the kind words, Viktor. Glad you're enjoying the videos!
Geiser would have actually worked had you installed geiser-guile and not geiser-mit...
Ya I realize that mistake later and fix it!
You actually had a memory leak in your fizzbuzz when you passed the heap allocator, you should've used a general-purpose allocator instead, and it would've given you an error
rare fellow emacs user spotted
how can you be a rust dev and never tried c++ before? That just makes no sense
I feel sorry for your clearly limited experience! Hope you have a much broader set of experiences in your future! ❤
As dismissive as this comment is, and even with the reply from OP being no better, it is an interesting thing to see. Clearly the knowledge of toolchains and lower-level language semantics are missing, and yet OP is able to write functioning rust code. You can check their repo for their initial rust implementation they use as a reference, and despite the project being simple and having some inconsistencies, it looks like the code is perfectly functional. For better or for worse, writing working code like this was not possible for OP in C++ and I think that does an interesting job of showing how approachable rust is compared to C++ when both languages produce pretty much the same results. I come from C++ also, and seeing the code being written here in C++ is painful ofc, but that's a problem caused by C++ and how non-approachable it is in regard to modern C++ and its differences from C++ 30 years ago. If rust is able to get through all this while being somewhat similar in capabilities to C++, I think that's a good thing.
Solid take@@LogicAtBest -- approachability is definitely a big Rust W despite the language having a reputation of being "hard to learn". I didn't put effort into a reply to the original dismissive comment because it was in fact dismissive ;). But there's a reason "Rust dev" is in quotes. I've learned Rust over the last few months with no systems programming background (just 10+ years off application development, primarily backend web development), and I don't have a CS degree. I appreciate your well thought out comment.
i had vague experiences with C++ before rust, but its definitely not somehow a prerequisite. C++ is like the final boss, not the tutorial boss.
Prepare for hours upon hours of configuration and Makefile madness 😢 Still C++ is just the main programming language for software and game development.
C++ has better options than a simple Makefile, CMake is pretty nice and easy to work it, and theres other options like Premake, Xmake etc, u also can deal with external libs with git submodules or using something like conan, bazel, vcpkg etc
this is cool
man, you are cool! Can we see some blockchain dev from you if it possible?) Will be great!
Not in the current plans but you never know ;)