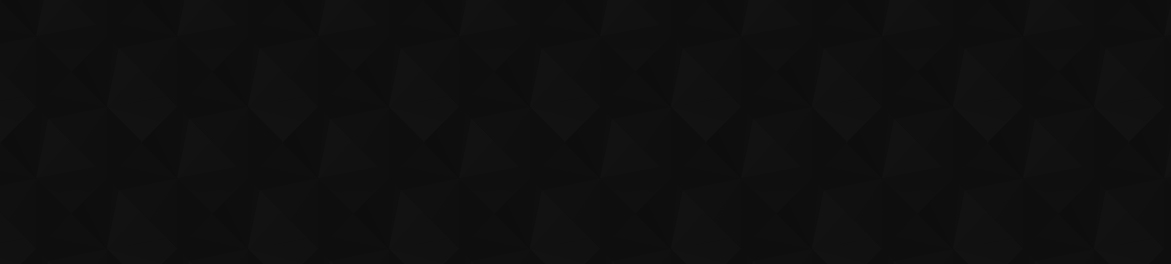
- Видео 15
- Просмотров 292 720
Making Sense Remotely
Германия
Добавлен 19 май 2020
Visualizing multi-band satellite images in Python
In this tutorial, I explain how to visualize multi-band Landsat 8 satellite imagery as true- and false-color composites using Python and matplotlib. The basic procedure is: 1) read individual raster bands as array 2) rescale to 0 and 1 using minimum and maximum values (or percentiles or mean + - standard deviation for contrast enhancement) 3) stack arrays using numpy.dstack 4) plot RGB composite with plt.imshow()
Code:
from osgeo import gdal
import numpy as np
import matplotlib.pyplot as plt
def scaleMinMax(x):
return((x - np.nanmin(x))/(np.nanmax(x) - np.nanmin(x)))
def scaleCCC(x):
return((x - np.nanpercentile(x, 2))/(np.nanpercentile(x, 98) - np.nanpercentile(x,2)))
def scaleStd(x):
return((...
Code:
from osgeo import gdal
import numpy as np
import matplotlib.pyplot as plt
def scaleMinMax(x):
return((x - np.nanmin(x))/(np.nanmax(x) - np.nanmin(x)))
def scaleCCC(x):
return((x - np.nanpercentile(x, 2))/(np.nanpercentile(x, 98) - np.nanpercentile(x,2)))
def scaleStd(x):
return((...
Просмотров: 12 508
Видео
Spatial Interpolation with GDAL in Python #2: IDW and Linear Interpolation
Просмотров 14 тыс.3 года назад
In this second interpolation tutorial, I talk about the Inverse Distance to a Power and Linear Interpolation algorithms available for the program gdal_grid (or gdal.Grid() if you use the GDAL Python API). I explain a little about the theoretical background of these spatial interpolation algorithms and then apply them to create a regular grid from scattered point data in Python. gdal_grid docume...
Spatial Interpolation with GDAL in Python #1: Nearest Neighbor and Moving Average
Просмотров 13 тыс.3 года назад
In this tutorial, I will give an introduction to the spatial interpolation algorithms nearest neighbor and moving average. We will use gdal.Grid() from the geospatial data abstraction library GDAL to create a regular grid from scattered point data in Python. gdal_grid documentation: gdal.org/programs/gdal_grid.html GDAL Grid Tutorial: gdal.org/tutorials/gdal_grid_tut.html#gdal-grid-tut GDAL/OGR...
Convert between CSV and GeoTIFF with GDAL in Python
Просмотров 20 тыс.3 года назад
Recently I got a couple of questions regarding the conversion of raster data from CSV to GeoTIFF and vice versa, so I decided to make a tutorial on this topic. In this video, I explain different methods to convert a GeoTIFF file into a three-column structure with X Y coordinates and raster value and to convert CSV data into GeoTiff using GDAL and Python. GDAL/OGR Python API: gdal.org/python/ Ch...
Merge raster data with GDAL in Python
Просмотров 17 тыс.3 года назад
This tutorial explains how to merge a bunch of raster tiles from the same directory to a single geotiff file using GDAL in Python. There are two options to merge raster data with GDAL: 1) run gdal_merge.py with subprocess 2) build a virtual mosaic of all raster files and convert that to geotiff. GDAL/OGR Python API: gdal.org/python/ Code: from osgeo import gdal import glob import subprocess # l...
Splitting raster data into equal pieces with GDAL in Python
Просмотров 11 тыс.3 года назад
In this tutorial, I will guide you through the creation of a Python script that uses GDAL to split raster data into several equal parts. The key to this task is to find out the xmin, ymin, xmax, and ymax coordinates of the smaller tiles and then use gdal.Warp or gdal.Translate to adjust the output boundaries of the input raster data. Be aware that the output tiles might have a slightly differen...
Reproject, resample and clip raster data with GDAL in Python
Просмотров 22 тыс.3 года назад
In this tutorial, I explain how to use gdalwarp in Python to reproject raster data to a different coordinate reference system, change the resolution (resample) and clip it to a shapefile. GDAL/OGR Python API: gdal.org/python/ Code: from osgeo import gdal import numpy as np import matplotlib.pyplot as plt ds = gdal.Open("dem.tif") # reproject dsReprj = gdal.Warp("demReprj.tif", ds, dstSRS = "EPS...
Processing DEMs with GDAL in Python
Просмотров 14 тыс.3 года назад
In this tutorial I explain various approaches to calculating terrain attributes such as the slope, aspect and hillshade of a digital elevation model (DEM) in Python. The different options are: 1) Run gdaldem with os.system() or subprocess.call() gdal.org/programs/gdaldem.html 2) Use the GDAL/OGR Python API and DEMProcessing() gdal.org/python/ 3) Use richdem.TerrainAttribute() richdem.readthedoc...
Read and write vector files with GDAL/OGR in Python
Просмотров 15 тыс.4 года назад
This tutorial explains how to open vector files with GDAL/OGR in Python, add attribute fields to store measures such as the perimeter and area of a polygon, and save the updated features to a new shapefile. We will also look at how to create a geometry from individual coordinates in Python and write it to a new vector file. GDAL/OGR Python API: gdal.org/python/ Python GDAL/OGR Cookbook: pcjeric...
Read and write raster files with GDAL in Python
Просмотров 45 тыс.4 года назад
This tutorial explains how to read raster data as an array and save arrays as a GeoTiff file using the GDAL library in Python. GDAL/OGR Python API: gdal.org/python/ Code: from osgeo import gdal import numpy as np import matplotlib.pyplot as plt # import ds = gdal.Open("dem.tif") gt = ds.GetGeoTransform() proj = ds.GetProjection() band = ds.GetRasterBand(1) array = band.ReadAsArray() plt.figure(...
GDAL Tutorial #5: Process vector data with GDAL/OGR
Просмотров 7 тыс.4 года назад
This tutorial provides an overview of the vector programs that exist in GDAL/OGR, specifically ogrinfo and ogr2ogr. We will use GDAL and the command line process and transform vector datasets. Information on the projection, extent, geometry, etc. of a shapefile can be retrieved using ogrinfo. To convert between different vector file formats and merge shapefiles we will use ogr2ogr. Finally we w...
GDAL Tutorial #4: Rasterize and Polygonize
Просмотров 12 тыс.4 года назад
This tutorial explains how to convert between raster and vector files using gdal_rasterize and gdal_polygonize from the command line. I provide several examples for raster/vector conversion, including how to pass attribute values to the output raster file, how to perform an attribute-based feature-selection and how to create a multi-band raster storing values from different poygons in different...
GDAL Tutorial #3: Raster calculation
Просмотров 11 тыс.4 года назад
In this tutorial, we will explore the GDAL command line raster calculator gdal_calc.py. gdal_calc is the equivalent to the raster calculator in QGIS and the expressions from this tutorial are easily transferrable. I provide three examples for the use of gdal_calc.py: 1) Masking pixel-values above a given threshold (e.g. to extract river networks). 2) Computation of the Normalized Difference Veg...
GDAL Tutorial #2: Converting, Resampling, Reprojecting, Clipping
Просмотров 27 тыс.4 года назад
This second GDAL tutorial explains the processing of geospatial raster data using GDAL and the command line interface. The following commands are introduced: - gdalinfo to obtain information on a given raster dataset - gdal_edit to manipulate this information - gdal_translate to convert between different raster formats and resample data to a different resolution - gdalwarp to change the coordin...
GDAL Tutorial #1: Introduction + Installation
Просмотров 52 тыс.4 года назад
The Geospatial Data Abstraction Library (GDAL) is a collection of tools that allow you to read, manipulate and write both raster and vector data using GDAL and the Simple Feature Library (OGR). This tutorial video is an introduction to the GDAL library, showing examples for more efficient processing of geospatial data using GDAL and also providing help for installing the library on Windows and ...
Amazing video, and love your accent. Muchas gracias!
Please provide the files as well so it wont be confusing to practice the same
Hi. It's a great tutorial. Would you mind to share the files you have used in the tutorial please 🙏❤
Extremely helpful! Please keep updating this series!💕
Data Set available?
This is amazing, thank you!
Hi, I'm wondering is it possible to provide the 2nd argument to the Grid() function, not using a "points.shp" file name, but some other format? I don't have a points.shp file, but only the x- and y- and z- coordinates. How do I provide those to the Grid() function?
From where will get dem.tif file?
How to rotet tif file actioly im trying open tiff file using python but i can able to load but if compated to global mapper image is looking like mirrer image kindly help me to solove this issue
Thank you for this tutorial! Very helpful.
Perfect explanation, thank you!
Hi many thanks for the video. Very interesting allthough I am not yet quite there with my project. Is there a way how to combine hillshading with colormap to show the terrain relief in way that the shading conveys a 3D effect while looking at a 2D map?
Great tutorial!! I'm stuck on this, and I was wondering if you could help me out. I have a coarse mesh coarse.tif and a fine mesh fine.tif. The extent of coarse.tif is larger and completely includes fine.tif. Both have different resolutions, but I want the points (pixels) of each to be "nested", giving precedence to the arrangement of points in the fine mesh. This means that starting from the fine mesh, I want to construct a coarse mesh where there is one coarse mesh point for every 4 fine mesh points. In other words, the square formed by every 4 fine mesh points has the corresponding coarse mesh point in the center. Once this coarse mesh is created, the average of the involved fine mesh points is considered in the points where both meshes overlap, and interpolation of the coarse mesh points is used outside the overlap. Is it possible by using gdalwarp? Thanks
Useful and Clear ! Thank You for this Great Tutorial
is there a way to have gdal working with react project.
Fantastic!!!
Can you please provide solution for gdal cropping RGB images into black and white patches
Hello, thank you for this tutorial it helped me a lot of, I just have a 1 question, I modfied your code instead of 1 data, I'm trying to divide folder of sentinel 2 images to 32x32 tiles, even though I don't get error and when printing it does show the coordinates of newly divided tiles, but output folder remains empty I think warp is not doing what it supposed to do and I don't know what else I could to do get it working, can you tell me what I'm doing wrong? This is my code import os from osgeo import gdal # Specify the input folder containing 256x256 images input_folder = r"E:\ytu\PHD\data eslihan_sentinel_testing\kodlar\SmartpolAIProcess\images" # Output folder for 32x32 tiles output_folder = r"E:\ytu\PHD\data eslihan_sentinel_testing\imagesTiles" image_files = [file for file in os.listdir(input_folder) if file.endswith('.tif')] # Loop through each image file for image_file in image_files: sen = gdal.Open(os.path.join(input_folder, image_file)) # Get the geotransform gt = sen.GetGeoTransform() # Get the dimensions of the image xlen = gt[1] * sen.RasterXSize ylen = gt[1] * sen.RasterYSize # Number of tiles in x and y direction div = 8 # Size of a single tile xsize = xlen // div ysize = ylen // div # Create lists of x and y coordinates xsteps = [gt[0] + xsize * i for i in range(div + 1)] ysteps = [gt[3] - ysize * i for i in range(div + 1)] # Loop over min and max x and y coordinates for i in range(div): for j in range(div): xmin = xsteps[i] xmax = xsteps[i + 1] ymax = ysteps[j] ymin = ysteps[j + 1] print("xmin: "+str(xmin)) print("xmax: "+str(xmax)) print("ymin: "+str(ymin)) print("ymax: "+str(ymax)) print(" ") # Use gdal warp to create 32x32 tiles gdal.Warp( os.path.join(output_folder, f"{os.path.splitext(image_file)[0]}_{i}_{j}.tif"), sen, outputBounds=(xmin, ymin, xmax, ymax), dstNodata=-9999, )
Clearly explained! Thank you very much. This is really helpful.
Graciaaaaas <3
Haw to install gedal in Jupiter notebook
Oh! one more thing: you operate with x and y - which one should be latitude and which one the longitude?
x is lon and y is lat, like in a map ... right?
@@ecoro_ thanks!
Bless you for covering different use cases! And thanks than mine is included.
Very useful and informative!
Please complete the gdal python series. Also it would be very much helpful if you could create a video on how to convert lat long ( spatial coordinates) to pixel coordinates (x,y) and vice versa, with introduction to different projection and crs. Thank you for the video though
Thank you for your videos! It's the third video that has been really helpful for me - appreciate it
my python version is >3.8 and its returning error to import gdal from osgeo what should I do
Great video! I've merged a couple of datasets with no issue, but now I get this error: ERROR 1: ZIPDecode:Decoding error at scanline 0 ERROR 1: TIFFReadEncodedTile() failed. ERROR 1: \\Path to this file...\DTM_1km_6159_636.tif, band 1: IReadBlock failed at X offset 0, Y offset 0: TIFFReadEncodedTile() failed. the data is from Denmark and I've got different offset values like x=6, 1 or 8 and y=5 or 6 I've been stuck on this problem for a while and tried all the solutions I've found on the internet, have you encountered this problem before?
I am facing difficulty while installing gdal. The error shows 'Environment Not Writable' Please help me.
Thanks for the tutorial, very awesome! Just wondering where to download your data for reproducing your demo? Thanks. 🙂
These tutorials are so incredibly helpful. Thank you!!!
Muchas gracias, clarísimo el video, entendí todo!
thanks
Thank you so much! I was looking to convert CSV to Tiff and none of other posts on internet seemed to work. Your tutorial was perfect solution to my use case. Thanks again!
I want to generate the raster data, so what should be the method i should follow should be it on python or any other ? Please suggest me some
I tested the code and I found two issues: 1) using gdal warp i got a Warning: "Warning 1: for band 1, destination nodata value has been clamped to 0, the original value being out of range." It seems related with the nodata values. At the end the resulting tiles are not workables. 2) the resolution res is not valid for y-axis, therefore the tiles generated are incomplete. I defined resx and resy (one per axis), at the result was ok. But in general, your code is the best example that I found surfing the web. Thanks a lot.
thank you so much
u're a lifesaver, thank you so much!
Thanks for all this, it helps a lot!
Come back pls!!!
Nice Tutor and Tutorial ! where can I get the sample data used in the tutorial? Thanks!
first of all congratulations for the content!!! would you know how to inform how the code would be to convert a raster (orthomosaic) tiff into ecw???
how can we do aspect analysis and slope analysis?
why when I run your code, it appears such errors : 'NoneType' object has no attribute 'GetRasterBand', could you explain it for me a while ! thanks!
Nice.
Is there any way to get access to the files you use here? I want to code along but don't have a tif that works like this one. Thanks!
Hey, Nice Video. Can I install GDAL library for MATLAB
Thank you for the video, How can I conver GeoTIFF to Binary?
can u help how can get image data or reflectance by using polygon shape file
Amazing tutorial thank sooo much!!