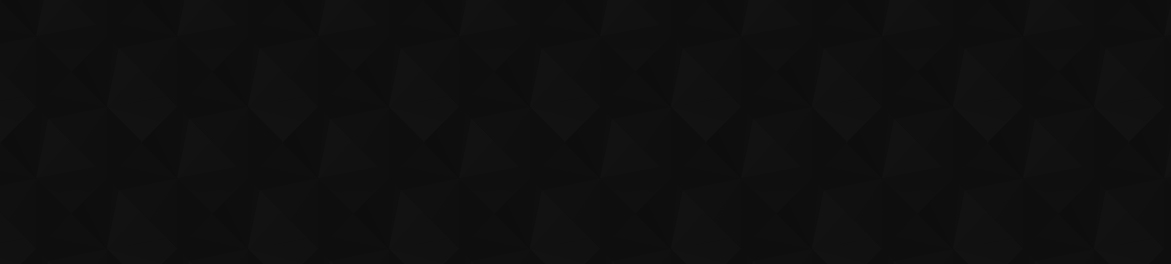
- Видео 33
- Просмотров 181 565
Everything C#
Австралия
Добавлен 20 мар 2015
Long-time software developer with extensive experience of C# and .NET.
Use the GitHub link below to get the source code for all the channel content.
Use the GitHub link below to get the source code for all the channel content.
Property name from lambda expression in C#
A common pattern in C# is to pass a property name by using a lambda expression as the parameter. This video shows how to extract that property name from the passed in lambda expression.
The crucial code you need is the following:-
var expression = (MemberExpression)action.Body;
string propertyName = expression.Member.Name;
Effective C# answers common questions about the C# language and the dotnet ecosystem. If you have a programming question about C# or .net then add it as a comment below.
GitHub: github.com/ComponentFactory/CSharpAnswers
The crucial code you need is the following:-
var expression = (MemberExpression)action.Body;
string propertyName = expression.Member.Name;
Effective C# answers common questions about the C# language and the dotnet ecosystem. If you have a programming question about C# or .net then add it as a comment below.
GitHub: github.com/ComponentFactory/CSharpAnswers
Просмотров: 738
Видео
Unleash the Full Potential of C# with These List Patterns
Просмотров 612Год назад
C# has introduced list patterns that allow for simpler, more concise and readable code. Combining existing patterns such as discard, property, logical and so forth makes pattern matching more powerful than ever.
Visual Studio Code and C#: Getting started using these 6 extensions
Просмотров 21 тыс.Год назад
To get the perfect setup for C# and Visual Studio Code you need the 6 extensions outlined in this video. Plus, an extra setting that few know but everyone should be using. 1: C# 2: C# Extensions 3: .NET Code Test Explorer 4: Rosylnator 5: Better Comments 6: CodeSnap
C# Syntactic Sugar: The Secret Ingredient
Просмотров 521Год назад
Syntactic sugar is the secret ingredient that enables the C# compiler to add new features without needing to make changes to the underlying tooling, such as the CLR, JIT, AOT compiler and others. Link: sharplab.io
Visual Studio Code vs Visual Studio
Просмотров 123 тыс.Год назад
Deciding on Visual Studio or Visual Studio Code is not just a personal decision but based on the best option for the operating system and application type you are building. VS Code: code.visualstudio.com/ Visual Code: visualstudio.microsoft.com/
Chunk your LINQ results in C#
Просмотров 394Год назад
Process your LINQ results in chunks by using this little known operator. Effective C# answers common questions about the C# language and the dotnet ecosystem. If you have a programming question about C# or .net then add it as a comment below. GitHub: github.com/ComponentFactory/CSharpAnswers
Add index to LINQ results in C#
Просмотров 342Год назад
You can add an index to the results of a LINQ query by using this simple trick. Effective C# answers common questions about the C# language and the dotnet ecosystem. If you have a programming question about C# or .net then add it as a comment below. GitHub: github.com/ComponentFactory/CSharpAnswers
Shuffle any list in C#
Просмотров 1 тыс.Год назад
This handy LINQ extension method provides any easy way to shuffle any IEnumerable list of items. For example, values for a deck of cards or any other type in your application, such as Unity. Effective C# answers common questions about the C# language and the dotnet ecosystem. If you have a programming question about C# or .NET then add it as a comment below. GitHub: github.com/ComponentFactory/...
Compiler internals for the record type in C#
Просмотров 187Год назад
See the code the compiler generates for record types to really understand how they work in C#. C# Answers provides answers to common questions about the C# language and the related .NET system. Do you have a programming question about C# or .NET that you would like answered? Add it as a comment below and I try to answer them all! GitHub: github.com/ComponentFactory/CSharpAnswers
Using IDisposable in C#
Просмотров 2,6 тыс.Год назад
Implement the IDisposable interface when you have managed or unmanaged resources that need cleaning up without waiting for the garbage collector to run. C# Answers provides answers to common questions about the C# language and the related .NET system. Do you have a programming question about C# or .NET that you would like answered? Add it as a comment below and I try to answer them all! Microso...
Paging results from LINQ in C#
Просмотров 418Год назад
You often need to add paging to a query so the application user can see the results in a table and then move page by page through the data. C# Answers provides answers to common questions about the C# language and the related .NET system. Do you have a programming question about C# or .NET that you would like answered? Add it as a comment below and I try to answer them all! GitHub: github.com/C...
GroupBy multiple properties in LINQ C#
Просмотров 2 тыс.Год назад
Sometimes you need to perform a LINQ GroupBy using more than a single property. This video will demo how to do this in C#. C# Answers provides answers to common questions about the C# language and the related .NET system. Do you have a programming question about C# or .NET that you would like answered? Add it as a comment below and I try to answer them all! GitHub: github.com/ComponentFactory/C...
Required keyword in C# 11
Просмотров 227Год назад
The 'required' keyword allows you to reduce the boilerplate code in your projects by using object initialization. C# Answers provides answers to common questions about the C# language and the related .NET system. Do you have a programming question about C# or .NET that you would like answered? Add it as a comment below and I try to answer them all! GitHub: github.com/ComponentFactory/CSharpAnswers
Dynamic LINQ queries in C#
Просмотров 4,2 тыс.Год назад
Sometimes you need to create a LINQ query that is dynamic and can be adapted to requirements such as responding to users clicking on different columns for sorting. C# Answers provides answers to common questions about the C# language and the related .NET system. Do you have a programming question about C# or .NET that you would like answered? Add it as a comment below and I try to answer them a...
Difference between IEnumerable and IQueryable in C#
Просмотров 2,6 тыс.Год назад
Many developers use LINQ without knowing the real difference between these two important interfaces. C# Answers provides answers to common questions about the C# language and the related .NET system. Do you have a programming question about C# or .NET that you would like answered? Add it as a comment below and I try to answer them all! GitHub: github.com/ComponentFactory/CSharpAnswers
Join using multiple properties in LINQ in C#
Просмотров 324Год назад
Join using multiple properties in LINQ in C#
Difference between Select and SelectMany in LINQ in C#
Просмотров 4,8 тыс.Год назад
Difference between Select and SelectMany in LINQ in C#
Multi property ordering, OrderBy, using LINQ in C#
Просмотров 544Год назад
Multi property ordering, OrderBy, using LINQ in C#
Differences between a field and a property in C#
Просмотров 4,3 тыс.Год назад
Differences between a field and a property in C#
Sort a generic List by a property of the objects in C#
Просмотров 5 тыс.Год назад
Sort a generic List by a property of the objects in C#
NullReferenceException and how do I fix it in C#
Просмотров 731Год назад
NullReferenceException and how do I fix it in C#
What do two question marks / null coalescing / '??' do in C#?
Просмотров 289Год назад
What do two question marks / null coalescing / '??' do in C#?
Best way to catch multiple exceptions in C#
Просмотров 212Год назад
Best way to catch multiple exceptions in C#
awesome
👏👏
I recognize your voice from Unity!
excellent video. straight to the point, how tutorials should bee
💯Best SelectMany explanation!! Thanks for all the many examples and code!!
I searched "Vs vs Vs code" and didnt expect to find anything, yet here i am! Great video.
my fellow human, u know nothing about internet if you think you will search something and you wouldn't find anything about it. there is something about EVERYTHING, on the internet.
@@atharvsharma1866I dont know, I don't get anything when I search "ajshejaksiejabakkdosjb ajajjanrls" on Google
Jetbrains!
useless ?.. saved my life atm.
Great
i adore how your speech is well-written
That was really helpful. Thanks man
Nice video!! Which one do you recommend for C developers? Thanks!
visual studio code
I'm linux user. Is writing code on c# and .NET in linux on VSCode painful thing? Should i download virtual machine to have visual studio on windows there?
what if there are two lists? a list with won years and a list with participated years. selectMany is executed consecutive? so there will be "stupid" duplicates? do you know of a way to achieve such without duplicates?
I want to integrate my my test cases from test plan in azure devops with the automated test cases from visual studio code/visual studio… so that where I automate them the test result in the test plan ado should change Is it possible?
Yes let's talk about it
Shouldn't it be private set { _age = Math.Min(120, Math.Max(0, value)); } ??
very helpful - hoping you'll be making more videos soon
good video.
Correct me if I err, but at 2:54, I believe you say "Creating the same project in Visual CODE is much simpler." Isn't this your intro to Visual BASIC, though? You've begun by showing us VBStudio, and now you want us to see Visual BASIC, right? I'm very confused by this.
Very Useful Thanks a bunch
the most useful video!
Totally waste of time 👎🏻
What makes this LINQ dynamic? Is it the switch statement?
What is OrderColumn? Is it from the Microsoft.SqlServer.Management.Smo ?
Thank you for your clear English and your time.
Can I open the code in vs 2022 that i writted in vs code
You don't even show how to actually use it. Useless!
What do you reccoment for flutter devs
good
Well, that was a short video for me.
you're transposing the names and probably don't realize it. FYI
Beautifully explained.
Sir please Reply to me 🙏I am a Student and trying to do Project of Music player in C++. But there is no option of "New Project" and "Console Application" in VS Code, am i Right Sir ? So that's why i should go with visual studio right ?
Vscode is a speedboat, visual studio is a yacht
You started off the video perfectly 😁
thanks for the explanation.
You did not mention what IDE to use on Macbook M chips.
This tip is amazing for dealing with multiline output string. Thanks a lot.
So both :)
thanks you. Great Video ^^
Well, there you go.. VS Code is great for writing "hello world" type programs that output text to a DOS window! Yay! Is this 1990 again? If you actually want forms and data grids and input boxes and buttons and to actually write a useful desktop program you can't do it with VS Code. I've been writing programs for Windows for many years and not once have I ever wanted to write text to a DOS window! What kind of childish program would I write with VS Code? Why would any serious programmer chose such a limiting program as VS Code over the much more useful tool of Visual Studio? (at least when it comes to Windows desktop programs) I can't believe anyone would recommend a tool that only generates text output with no forms and no display tools.
Microsoft should come up with a standalome xaml builder or form builder. I wonder why they don't. its as if we are forced to use visual studio 😅
intro was interesting
Thanks for the explanation :)
You just solve my millions of years confusion.
I can relate. I was following a course using visual studio when they were using visual studio code. I couldn't find the ejs plugin so here I am. uninstalling vs and installing vs code now
reupload video, please
Excellent explanation
Thank you for this
I have developed an app with vs code but I caanot find extension to create installer. What am I missing?
awesome explanation ❤
Just use VS code in the end... its better... i am a hobby programmer.. i am still learning but im getting there... my language of choice is Lua... and VS code supported is out of the box from what it seemed like and others... so thats nice