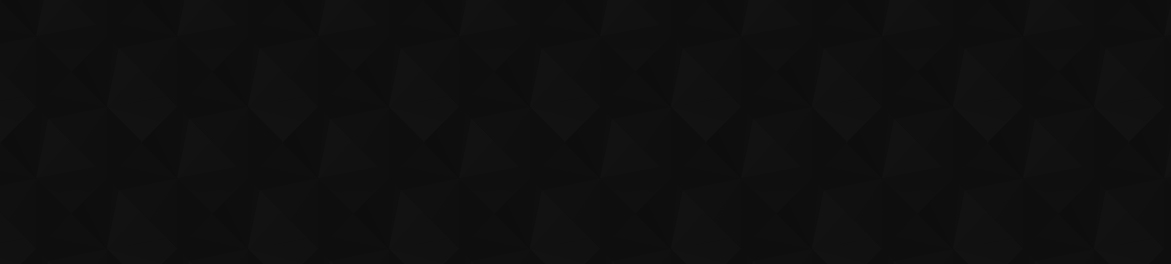
- Видео 45
- Просмотров 107 472
Code for yourself
Швейцария
Добавлен 15 янв 2022
This channel is all about code. After more than a decade-long career in programming and designing software and a PhD in robotics and computer vision, I decided to have my own place on the Internet where I can chat about things I encountered throughout the years. The content will have more or less rigid courses on that or another programming language or technique, but might eventually morph into something less structured. Only time will tell. For now - welcome to this new journey and hope you will enjoy it with me!
INHERITANCE in C++ | most of the things you need to know for OOP and beyond 🚀
In this video we cover most of the things that one might encounter when working with inheritance in modern C++. We go over a broad list of topics, starting from why we care about inheritance (spoiler alert: to enable dynamic polymorphism) and digging down into the rabbit hole of how it mixes with implementation inheritance (not that well), what is object slicing and what we need to keep in mind should we want to use inheritance and embrace reference semantics.
🙏 *Support what I do*
If you believe that free education is a good thing, you would like to see more of it, and you like my way of teaching C++, please consider supporting my efforts. I choose to not hide this course behind a paywall ...
🙏 *Support what I do*
If you believe that free education is a good thing, you would like to see more of it, and you like my way of teaching C++, please consider supporting my efforts. I choose to not hide this course behind a paywall ...
Просмотров: 986
Видео
Separate declaration and definition for C++ templates? Yes please (sometimes)
Просмотров 1,4 тыс.Месяц назад
It seems that the topic of compiling templated code in C into libraries is one of the typical contention topics for lots of people when they use templates in their code. In this video we look into why this topic can be perceived as such a complex one as well as how we can actually achieve what we want. 📚 As always, you can find the full script to this video as well as all the code shown on the ...
Why use forwarding references and how they are different from rvalue references in C++
Просмотров 1,8 тыс.2 месяца назад
Since C 11 was released there has always been quite some interest in forwarding references among people practicing C . On one hand, it is a very powerful mechanism, on the other, it requires quite some knowledge about how templates work so it is met with a lot of fear among people learning C . In this video, we cover everything there is to know about forwarding references, why we might want to ...
How to use CLASS TEMPLATES, type traits, partial and full class template specialization
Просмотров 9123 месяца назад
Class templates with the ability to partially and fully specialize them are arguably what makes C so powerful! We cover most of what one needs to know about what class templates are, how to specialize them fully or partially as well as how it all plays its role if we want to implement type traits. Before you watch this video I recommend you to watch these videos if you haven't already: - *why* ...
How to use FUNCTION TEMPLATES - a comprehensive guide for modern C++
Просмотров 2,3 тыс.5 месяцев назад
Writing function templates is part of the core skills we can use as a C programmer! Today, we cover close to everything there is to know about this topic skipping only very few cases that we leave for the future. Before you watch this video I recommend you to watch these videos if you haven't already: - *why* use templates: ruclips.net/video/1Mrt1NM3KnI/видео.html - *what* templates do under th...
Templates under the hood
Просмотров 1,2 тыс.6 месяцев назад
The second video out of a number of videos about templates. Here we focus on *what* templates do under the hood. Or, more precisely what a compiler does when it sees templated code. If you want to know *why* use templates in the first place, see: ruclips.net/video/1Mrt1NM3KnI/видео.html 📚 As always, the script to the video lives here: github.com/cpp-for-yourself/supplementary-materials/blob/mai...
Why use templates in modern C++
Просмотров 1,9 тыс.7 месяцев назад
The first video out of a number of videos about templates. Here we focus on *why* anybody would want to use templates in the first place, leaving the details to what templates do and how to properly use them in C to the future videos. If you want to know *what* templates do under the hood, see this video: ruclips.net/video/NKvEbPVllRE/видео.html 📚 As always, the script to the video lives here: ...
Use static in classes (when needed) in modern C++
Просмотров 1,4 тыс.9 месяцев назад
Everything that you wanted to know about static (when it is used *inside* of classes) but were afraid to ask. For when (not) to use static *outside* of classes, see ruclips.net/video/7cpPQunjv4s/видео.html 📚 As always, the script to the video lives here: github.com/cpp-for-yourself/supplementary-materials/blob/main/lectures/static_in_classes.md 00:00 - Intro 01:26 - Static class methods 04:54 -...
Don't use static (outside of classes) in modern C++
Просмотров 5 тыс.9 месяцев назад
Everything that you wanted to know about static (when it is used outside of classes) but were afraid to ask. The video on using static *in* classes can be found here: ruclips.net/video/ggNCjDPShrA/видео.html UPD: Had to re-record and re-upload due to some issues with the previous attempt at this video. 📚 As always, the script to the video lives here: github.com/cpp-for-yourself/supplementary-ma...
Const correctness in C++
Просмотров 3 тыс.11 месяцев назад
Here we look at const correctness as one of the cornerstone parts of C . We already know how to write our functions and classes and have access to all use cases where we can and want to use const keyword. So we cover most of these use cases here. 📚 As always, the script to the video lives here: github.com/cpp-for-yourself/supplementary-materials/blob/main/lectures/const_correctness.md
Project: Pixelate Images in Terminal 😎
Просмотров 1,3 тыс.Год назад
We've learnt a lot until now. We know how to write classes, how to implement proper move semantics for them as well as how to create full projects with CMake. Now it is time to put it all to a test by implementing a project that allows us to load an image from disk, pixelate it and show it to the user in the terminal. 📚 As always, the script to the project description lives here: github.com/cpp...
Headers and libraries, but with classes
Просмотров 1,5 тыс.Год назад
We know how to write libraries when using just functions. Is it any different when we use classes? We'll figure out in this video! Turns out the situation is nearly the same as when not using classes with just a couple minor differences. 📚 As always, the script to the video lives here: github.com/cpp-for-yourself/supplementary-materials/blob/main/lectures/headers_with_classes.md The chatbot use...
Safely copying, moving, and destroying objects in Modern C++ with the rule of "all or nothing"
Просмотров 2,2 тыс.Год назад
Which constructors does a C class need? Which does it generates on its own? What about various assignment operators? And a destructor? How not to shoot ones leg off and navigate these waters effortlessly? This and more we cover in this video that tells us a simple rule to follow when implementing classes in Modern C , the rule of "all or nothing". We dive into the reasons for its existence and ...
Re-inventing move semantics in modern C++ in 13 minutes
Просмотров 6 тыс.Год назад
Move semantics, value semantics, rvalue references, rvalues, lvalues etc. are all terms related to what lies at the heart of modern C . These concepts are all related and in this video we look at exactly what they mean. By re-inventing them. In this video we re-invent move semantics as it exists in C 11 starting from the reasons for its existence and inventing every single machinery that is use...
C++ object lifecycle 101: the essentials
Просмотров 1 тыс.Год назад
We regularly create instances of objects in C . These objects get created and destroyed. Today we learn the basics behind how it all happens through the machinery of constructors and destructors. We will cover when which constructor is called, what are member initializer lists, when to use explicit keyword and what 📚 As always, the script to the video lives here: github.com/cpp-for-yourself/sup...
Mastering C++ pointers: the pros and cons of (raw) pointers | pointer usage with const
Просмотров 1,6 тыс.Год назад
Mastering C pointers: the pros and cons of (raw) pointers | pointer usage with const
Can you write a proper CMake library? | homework 📚
Просмотров 1,6 тыс.Год назад
Can you write a proper CMake library? | homework 📚
Do you even test? (your code with CMake)
Просмотров 21 тыс.Год назад
Do you even test? (your code with CMake)
🏗️ Build your libraries easier using build systems
Просмотров 1,5 тыс.Год назад
🏗️ Build your libraries easier using build systems
C++ libraries and what inline has to do with them
Просмотров 3,5 тыс.Год назад
C libraries and what inline has to do with them
Functions in modern C++ and some related best practices 👌
Просмотров 1,3 тыс.Год назад
Functions in modern C and some related best practices 👌
Common compilation flags and debugging a C++ program
Просмотров 1,5 тыс.Год назад
Common compilation flags and debugging a C program
what fonts you use in terminal? i liked them
I’m not entirely sure which one I used back then but I have mostly converged on using Fira Code everywhere now.
I like your (semantic) separation of impl.. inheritance and polymorphic inheritance 🎉
@@bsdooby thanks! I think it makes sense to teach them as separate concepts altogether
Fantastic explanations and visuals!
Thanks a lot for the compliments! 😌
Nice video! Why is the int* 8 bytes instead of 4 at 1:30?
@@Pedro-jj7gp that’s just the way it usually is on most modern systems: any pointer is 8 bytes. In the end this has to do with being able to address enough places in the memory if this makes any sense. That being said, other systems could theoretically have it be smaller or larger. Does this answer your question?
@@CodeForYourself It does, but I'm a bit confused as I was watching some other videos about vtables and they showed the vpointer taking up 4 bytes... I guess it could just be on that system.
@@Pedro-jj7gp it might be. But you can always check it on your system too. I’ve only ever seen 8-byte pointers on every system apart from really non-standard embedded boards. 🤷♂️
@@CodeForYourself Yup, you're right! I just checked using sizeof() both for a regular int* and a vpointer using reinterpret_cast<int*>() and both are 8 bytes. The video in question was 12 years old, maybe it was a 32-bit machine
@@Pedro-jj7gp sounds like a 32 bit system indeed. Glad that it makes sense now 👌
Nice and clean overview. However, dynamic polymorphism is a very well known and old topic in C++. I would suggest deepening into static polymorphism for the next video. Keep it up, it is refreshing to see technical C++ stuff in RUclips 2024
@@SystemSigma_ thanks! I actually put static polymorphism before this video, it’s right along a series of videos about templates: ruclips.net/video/1Mrt1NM3KnI/видео.html Still not the most modern thing but necessary to understand concepts down the line I believe. I also wanted to give an overview eventually of how to do the same things using both dynamic and static polymorphism on some concrete examples. But for that people need to be familiar with both. Does this make any sense?
@@CodeForYourself It does indeed. I meant that modern c++ features (especially type traits) are really useful for making more robust static polymorphic code. Maybe instead of going too generic with topics, restricting the features scope may be more interesting for experienced viewers.
@@SystemSigma_ I agree that a more in-depth look would suit experienced folk better. My aim here, however, is to fill in the complete playlist that would tailor to somebody who knows nothing about C++ and guides them gently through all the main topics, providing the "why" for using what they learn along with enough information to dig deeper. Please see here for the full list to date: github.com/cpp-for-yourself/lectures-and-homeworks Maybe one day, I will finish the basic course and have some time to dig more in-depth into certain things. That being said, I feel that there is plenty of that type of content on RUclips already, while the comprehensive courses that I've seen all fell a bit short of what I wanted to achieve here. But we'll see if my course is going to be helpful to anybody 😅
@@SystemSigma_ oh, and just in case you have not seen already, I _do_ talk about type traits a bit more in-depth here: ruclips.net/video/IQ62tA51Vag/видео.html
Hi. I think I have the C++ part of this completed, and would like to submit it for testing. But I'm a bit lost on the git side of this. I tried to use the same git template instance, and put my fortune_celler.cpp in ~/src/Full-C++17-course/t/cpp-for-yourself/homeworks/fortune_teller/task in a fresh branch (I also tried it in the same branch), but upon push'ing that to origin, I just got the example_homework graded again. Any suggestions?
Thanks for the video. Please, can you focus more on Modern C++. Here are some: 1. Coroutine 2. Multithreading/Concurrency 3. Testing
@@McDonaldIbekwe ok, so there is one video on testing that I did before but it is not in-depth. As for coroutines I would have to use them in some real projects to be able to speak confidently about best practices that relate to them unfortunately. 🤷♂️ I will try to squeeze some multithreading in though at some point but I can’t say when just now 🤷♂️
structs do not need "public" inheritance; structs are public by default (sorry for being a PITA); you spare a few keystrokes ;)
Yes, you are totally right, but I much prefer to be explicit rather than implicit. My logic is that it is not a big deal to type one more word but it allows us to not think about which inheritance is going to be picked by the compiler implicitly should we omit that “public”. Does this logic make sense?
Чудова лекція. В майбутньому було б цікаво почути щось на тему concurrency, threads загалом.
Дякую! Радий, що сподобалось! Подивимось, взагалі план був про це розказати, але це така велика тема і в ній так багато чого відбувається, що я не впевнений що зможу її просто і добре пояснити. Але вона у мене в планах. 👍
nitpicking: if you use UML for you examples, the impl. arrow (realization of an interface) has a non-filled arrow head.
Yeah, I thought of fitting to the standard UML style but then left it at that considering that the tools I used for creating the visuals did not have a non-filled arrow 😅
SPOILER: Wrapping everything up with the Image class example from the start was a very nice move 👍 I really enjoyed this lecture and will definitely recommend this further. Thanks a lot for your awesome content! 👏
Thanks! I’m really happy that that move clicked. It was a long video so I wanted to end at one consistent example that would be relatively close to real life. 🙏
Haven't watched this yet, but I know it is good! One of the best C++ creators out there for sure!
Thanks for your support and such a trust in me 🙏 But feel free to revise once watched 😉
true words
This video took A LOT of time to make. Just the script I had to rewrite like 3 times. So, if you like what you see, please share this video with your C++ inclined friends if you found this video useful. 🙏 And do leave a comment, this way this video will be shown to more people apparently 🤷😉
Pure joy. Thanks!
@@GeorgiosMATZARAPIS thanks man 🙌
You have a very friendly face 🌞. I imagine it would be difficult for you to intimidate someone or to scold children
@@akshaypratap4123 that’s not how I think I am usually perceived at all. Especially when I’m coding. 😅
Could use dockerfile also to preinstall all gtest/grpc libs
Best explanation ever found for cmake
@@rishiniranjan1746 thanks! This means so much to me! 🙌
I am new to C++ templates and ran into this exact issue about an hour ago. This video helped me a lot, thanks!
@@BISONBOT94 awesome! Glad I could help out! 🙏
I wonder how the templates are used in data structures in STL (for ex: vector) so that it not only works with primitive data types like int, float,etc. (for which can do the template specialization) but also with user defined classes/structs, which is not known before hand. Any ideas ? @CodeForYourself
@@UvUtkarsh generally the code is written in such a way that as long as the provided types follow some form of an interface they should work. They also use traits and partially specialize classes based on what those traits tell about the provided type. I might do an in-depth look into this later if there is interest
what would you do with template class
There is an example of this towards the end of the video. Does that answer your question? Or should I give a better example? How can I make it better?
@@isfandyar3937 also, I cover this extensively in this video: Why use templates in modern C++ ruclips.net/video/1Mrt1NM3KnI/видео.html
Great explanation. Thank you
Glad you liked it! 🙏
If I'm not mistaken, this only helps speeding up compilation times due to the explicit template specialisation. Still, what other benefits do I get by splitting template declarations and definitions?
I guess you're right to a degree but there is more to it. Explicit template instantiation (different from specialization) does indeed help the compile times. It helps because without it, the code would live in header files and so would be copied to any translation unit that includes that header, leading to the compiler needing to compile all of those instances on their own. If we put the code into a source file (and for that we need an explicit template instantiation) we will only compile the code once and link it to the places where it is used instead. Does this make any sense? Now as to other benefits, one big downside of header-only libraries, apart from compilation times, is that all the implementation actually lives within the header files. Meaning that if we want to distribute our library to other people we essentially have to distribute source code. So in the end, we have a sort of a tradeoff, header-only libraries are quite simple to use but might lead to long compile times as well as to needing to show our source code to anybody using our library. I talk about this at length in the video about libraries here: ruclips.net/video/Lxo8ftglwXE/видео.htmlsi=JYNvd_2i6GLjfdvv
I'm perfectly aware of the standard benefits of splitting declaration and definition.. Still, for templates I really don't think it's worth the typing effort (if you're outside a smart ide) 😅 I guess the most valid point is only hiding the source code for distribution 😊
@SystemSigma_ sometimes the compile times are important too. Some years ago I added a couple of explicit instantiations in a code base to save about an hour compile time 😅 I think if we *know* the types we’re about to use, then I would go for a compiled library as opposed to a header only one. It works also as an additional check for my design to a degree 🤷♂️
@CodeForYourself yeah, I used it with quite a success in a few embedded projects, but, of course, every project is different so 🥲
@@SystemSigma_ yeah, I agree. 🙂↕️
Thanks a lot for watching, folks! Don't forget to share any thoughts you might have on this (or anything else)! 🙏
CMake Deez nuts
Great video! One thing that bothered me a bit was usage of "<typename ClassT> to specify template parameter. The parameter has nothing to do with class, it could be just as easily called <typename T>, the word "class" gives a wrong impression, at least to me. Thanks!
Ok, I agree with you that ClassT doesn’t hit the mark. What I prefer doing generally (when I cannot use concepts) is to give the template parameters meaningful names. Considering that this function is very illustrative and doesn’t really have a proper purpose it is hard to pick such a template parameter name, meaning the one that actually makes sense. So here I picked the ClassT as a substitute but I agree that it is a bit stupid and if I would have thought longer about it I probably would have just used T here. Thanks for your comment!
For the win, my man
@10:57 when we call DoSmth(number) i didn't expect the parameter type to be int&. Why is that, and not simply int? After all, it will be copied...
Unfortunately the answer to this comment is more complicated than I would like it to be. It has to do with how overload resolution is taken care of in C++, which is not trivial: en.cppreference.com/w/cpp/language/overload_resolution But the rule of thumb, at least the way I think about it, is that if we have a local object the compiler will prefer passing it by reference if an appropriate function overload exists. During this some implicit conversions can take place as well as some copies performed. My recommendation would be to write a simple example with some Foo function that has various overloads and see what the compiler does in each case to build more intuition. That being said if somebody who can explain it better stumbles upon this comment, I would like to find a nice and simple explanation for the future. 😅
@@CodeForYourself thank you, i really appreciate the answer, it surely provides a good starting point for me to try and understand this :) Keep up the great work, i discovered your channel with this video, and decided to start the whole course from the first lesson, even if I'm familiar with some of the basics of C++
@@francescobenacci6611 thanks for the kind words! Please mind that the first videos have a slightly different style from this one. I guess I converged to a style like this some time around the cmake video 😅
Universal Reference gang member Here, Scott Myers most loyal soldier.
Welcome 😅
An interesting fact is that forwarding (or universal) references were discovered and not designed in the language. They just happened to spring into existence following the rules of template deduction and reference collapsing. It's one of the many miracles of the language showing that when you design something right it gives more value than designer has originally anticipated. It's an example of 1+1 giving more than 2.
Yes, thanks for this comment! I believe the reason the name “universal reference” exists is just for that reason too! It came before the “forwarding reference” name was used in the standard. That being said, I don’t have the hard facts for this, so if somebody can point me in the right direction I would be immensely grateful 😉
Great Video. In the future would love to see Map with Object of class as key and as value. Love the music at the end. Please, can I get the full song?
Thanks 🙏 I’ll note the interest and we will see if I get to record something more about the map class. As for the music it is one of the RUclips samples. I’ll try to find the actual name tomorrow and send it your way.
I thought you'd say FetchContent, but submodule it is 🥳🥳
still can't figure out on what paramterts to put to make it choose this function T&& forward(std::remove_reference_t<T>&& t) instead
Did you try the ones at the end of the lecture? Like pass a real temporary value into the forward function directly?
@@CodeForYourself oh lol, my bad. I mistakenly put real temporary value into doSmth instead of forward. lol, i'm so stupid. sorry for bothering u.
@ilovenirajan no worries at all! This is what learning is all about 👌 I make these mistakes all the time!
I was not able to replicate the uninitialiazed variable problem in @22:00 it always showed 0.0000e+00
That is expected and is kind of the point that I was trying to make! Undefined behavior is like that: one cannot rely on it failing reliably. It will sometimes fail but then will also not fail at other time. Which makes it really frustrating 😬
@@CodeForYourself Thank you very much, Yes, I understand your point. I learned this the hard way once and now I have this uncontrollable urge of explicitly initializing everything (also usually this is the first thing I check when debugging) .
@en_x same on my side. It really pays great dividends! 😉
Thank you for walking through how this forwarding works, particularly the ampersand collapsing. I hadn't fully understood how forwarding works until you put it all together like that.
Thanks for the kind words! I have to admit that before recording the video I didn’t understand it fully either 😅 Well, I had a pretty good idea but then my intuition was wrong with respect to which forward overload would be called. 😅
You have some of the best videos on C++. I would love if after your "C++ basics" lessons that are happening, you could move to a more specialized subject. I see you have a PhD in computer vision, so that would be a cool subject to tackle in C++ (as a lot of tutorials use Python)
Thanks 🙏 I keep playing with this idea. I come from a robotics background and we’ll see if I manage to make something out of it once I finish the C++ course here 😅
I find it better to understand if I read `&&` as `owned` or `owned_value`. #define owned && So the difference between `int` and `int owned` is the ownership.
That’s an interesting idea, although I don’t like the use of macros here (what if you have a variable called owned in the code or smth) But apart from that it sounds fun. I’m not sure how I feel about it just yet as in my head a cope of a variable owns its memory too and can transfer it if we pass it through an std::move and some move assignment operator 🤔
@@CodeForYourself Yes, I do not use in code but for explaining and understanding it is good. You can show a `int &&` and then show `int owned` to compare it in different contexed. For example: Saying `int &&` / `int owned` has more in common with `int`, than `int &`. Comparing && and & can be very confusing.
Hey everyone. How did you find this video? Too long? Or short? Too complicated? Or rather too dumbed down? I need your feedback to make sure I’m getting better. So… what do you think? 🙏
I don't know any way you could cover this topic with sufficient detail and be any shorter or less complicated. For me, it was just right.
Awesome! Thanks for the feedback and glad you liked it! 🙏
You should give more time to read and understand the examples. It's really nice to see you talking, but often you return from code to yourself way too quickly.
I don't have much use for std::forward (and had no idea how it works, and when to use it), so for me, it had the correct amount of detail.
@@taschenorakel totally makes sense. In this format I made a deliberate decision of keeping up with the pace to keep people a bit more engaged. My reasoning was that if people would really want to return and follow all the details they can always pause the video. I might convert all of this into a proper course on a different platform geared for just learning where I could maybe reduce rhetoric pace somewhat 🤔 Thoughts?
My bet is: //2 //1 //3 //4 and 0 2 1 1
Can i also ask HTML or is it only C++?
I’m not an expect in web dev, sorry 🤷♂️😁 For now it’s just C++. But once I’m done with the course we’ll see what comes next. That being said, it’s more likely to be Rust rather than anything web oriented 🤷♂️
VERY USEFULL!!! thanks for making learning fun!
Glad you like it! 🙏
@@CodeForYourself im exploring your vids and they are really cool 😎💪
@D1eg0_ really glad to hear that! Don’t forget to tell your friends! 😬
Hi, I seem to forget small details like flags -c to not use linker etc. How to remember them?
I wouldn’t bother. It’s really not such a big deal. One usually needs those flags only to figure out some weird thing happening. Once you see the linker errors you know you forgot the -c flag 😁
What if we make header-only libraries instead.
You mean like not ever use any other libraries?
@@CodeForYourself okay I'm too much of a beginner. I think I understand the tradeoffs of different types of libraries. Header only libs makes the devs life simple. But if you want to hide the implementation then they wont work.
@@ChimiChanga1337 yes, you are exactly right! Also, header only libraries, when we have many of them, make compilation waaay too slow. With compiler libraries we are able to often compile them once and link all over the place. Does this make sense?
@@CodeForYourself Yes, I understand now. I really want to thank you again for this course!
@@ChimiChanga1337 my pleasure and I'm really happy that you like it! 🙏
Template meta programming with (partial) specialization is such a wildly different language than to "regular" C++. And it's such an incredibly deep rabbit hole. Interestingly enough, my knowledge about this topic really helped to understand Haskell a lot better during the semester it has been taught in. The signature pattern matching in partial specializations is almost identical to how Haskell functions are overloaded for their use cases. C++ really helps to become a better programmer, because it exposes the user to a lot more concepts than certain other languages that deem certain mechanisms to low-level or dangerous to be exposed to the programmer. But seeing how C++ was helpful for such an unrelated language with very different paradigms was just wild. :D
Thanks for sharing! Did not think of this parallel! I have only played a little with functional language but yes, the recursiveness of template meta programming I guess is what makes the experiences similar.
I came back to c++ after spending some time with ocaml, rust, and idris, and everything was so much easier. This template stuff makes a lot more sense now and these videos are really good
@harleyspeedthrust4013 thank you so much! Hope you keep enjoying the videos coming next! 🙌
Nice T-short, man!
Thanks, Dima 😉
Sir. You Can Make A Video C++ Virtual Tabes Dynamic Linking How Works. With assembly.
Not sure I want to include assembly in that video but otherwise the vtable video is planned already! 😉
@@CodeForYourself Just Waiting 🙋
Will try my best to make it as soon as I can 👌
BRUH. The universe might actually be alive. I have been struggling to understand templates and was a bit sad that you didn't drop a video on them. Now here you are. FTW.
Hope it helps 😅
That pretty much helpful and so easy to understand, thank you!
Cmake is absolute dogshit
While it has its quirks, this is probably too strong of a statement 🤷♂️
Does this code at the end of each test run attempt to update submodules? Will this slow down the testing process if tests are run frequently?
Thanks for the question! The update code is only run at the cmake configuration stage. So it is not run when the tests are run. Does this make sense?
Hi Igor, I am using CMAKE 3.16, when I tried the above I am getting a message stating that no tests found. How to resolve this?
Yeah, that’s a bit of a limitation. There was another comment under this video where we come up with a solution. Does that solution work for you?
@@CodeForYourself where is that test folder is mapped? I couldn't find it.
@whac-a-robot8623 it should be just the build folder itself. So you have to change the directory into that build folder and run the cmake command from there. If that doesn’t work out I’ll have a look more deeply into this.
@@CodeForYourself ok will check and let you know
@@CodeForYourself I understood it now. For quick reference for others you putting my solution here. Command 1 : cd build . Command 2 : GTEST_COLOR=1 ctest --output-on-failure -j12. Thank you for the support.
Really great video and I love how much you went through in such a short amount of time. I was messing around with code to produce the two problems with template specializations and I found some interesting behavior I was wondering if you could shed some light on. For the record, I was using C++23 and Clang. The code I tested first for the problem that template's will never match reference types unless they are explicitely specified worked exactly the way you showed. However, I tried the Dimov/Abrahams problem using an Object pointer instead of an int pointer becuase I had created an object class for testing the previous example. Everything worked like I observed in your video until I tried changing the specialization to a function overload. My code still picked the template-function overload instead of the function overload! This was really confusing to me since I thought regular functions were always preferred over templates. I even tried moving the functions around, but no order I put them in changed the result. This stumpted me to no end, I tried the same example with an int and it worked just like you said it would! It seems the rules for templates are different for object pointers for some reason? I then thought that maybe the fact that I had these functions in a header file I was including was contributing to the problem because of course they were marked inline when necessary, so I tried in my main file and I got exactly the same behavior. This actually only ended up confusing me more because I encountered more strange behavior I wasn't expecting regarding the namespace from my header file. As usual, I put the namespace name in front of all my function calls since I didn't declare that I was using that namespace. CLion said that the namespace name wasn't necessary on the function calls where I specifcally passed an object or the address of an object, but it was necessary on my function calls where I was passing in an int or the address of an int. I then tried running my code and lo and behold I got errors when removing the namespace on my primitive function calls but I didn't when I did it for my object calls! I have absolutely no idea what was going on here and I would love an answer. It got weirder yet again because I had functions with the same name in both my main file and my header file, but I didn't get an error when omiting the namespace specifically on the calls where I was passing an object or the address of an object, which was strange because I thought there would have been a name conflict, but even worse, C++ actually picked the function from the header file in a different namespace instead of the one in the current namespace! I've seen some bizarre things in C++, but this is one of the most bizarre and I'm completely lost. I had to use the global-namespace specifier to get C++ to choose the function from it's current namespace which didn't make any sense. Even weirder still, this was only a problem when I called the function with an object. When I called the function with the object's address, the correct one was picked! I know you're probably busy, but I would love it if you could take the time to answer or direct me to a video explaining it if you know of one! Here is the link: godbolt.org/z/sdGsWra8G.
Sounds interesting. I’d like to give it a go. Can I ask you to setup a small reproducer on compiler explorer and share the link here?
@@CodeForYourself I just realized today that RUclips deleted my reply because I guess it thought the link was spam. I'll try to do my best to get it to go through. I can't give you the full link, but if you go to the normal url for Compiler Explorer, it's a '/z/' then sdGsWra8G. Let me know if that works! Thank you for taking the time!
@@CodeForYourself I'm sorry it took me so long to respond! I thought I had, but it seems RUclips won't let me reply with the link. It actually kept deleting my reply! I just edited my original comment to include the link at the bottom. Thank you, again, for taking the time!
@@Awesomekid2283 thanks a lot. Got it. Will have a look soon!
@@Awesomekid2283 Ok, I've looked into your example and see a couple of issues that could cause the behavior you describe. First of all, your functions are all `inline` in the header. Which means that they already have all of their definitions. You don't need to (and generally cannot, but see the next issue point) redefine such `inline` functions in the cpp file. You are only able to do it because of the namespaces that you use. Which brings us to the second point. Second, you have different namespaces in the header file and the source file. All of the functions in the header file are in the `templates` namespace, while all of the functions in the cpp file are in the global namespace. Now what happens is this: the functions in the header file are fully defined. The functions in the cpp file *do not define previously declared functions, they define new functions in the global namespace*. This causes all of the issues that you experience. Does this make sense? If you try to add the namespace `templates` in your cpp file, you will get quite a few "redefinition" errors during the compilation. I would generally recommend to try to simplify your testing setups. I do it myself. If I want to make sure that I understand a certain concept, like template specialization, I build up a *minimal* example - as small as I can, to make sure I'm only testing what I wanted to. In your case, you have at least three things going on in the same time - header/source file separation, namespaces and then template specialization/overloading. Separating these into separate small examples lets us learn them more easily.