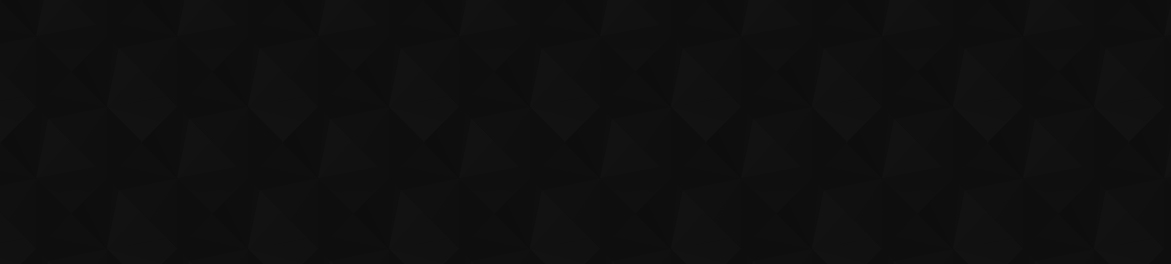
- Видео 183
- Просмотров 7 166
Code With K5KC
Германия
Добавлен 18 авг 2020
Welcome to Code with K5KC!
Dive into the ultimate destination for all things coding interviews and beyond. Our channel is dedicated to providing high-quality, comprehensive tutorials and insights that will help you ace your coding interview and excel in your tech career.
Learn from basic to advance coding skills and become a better programmer:
- Datastructure and Algorithms
- System Design
- Programming Languages like Java, Python, and more
And Much More: Stay tuned for a variety of content that will take your coding skills to the next level.
Whether you're prepping for an upcoming interview or looking to broaden your programming knowledge, Code with K5KC is here to support you every step of the way. Subscribe and join our community of learners and future tech leaders! 🚀
Dive into the ultimate destination for all things coding interviews and beyond. Our channel is dedicated to providing high-quality, comprehensive tutorials and insights that will help you ace your coding interview and excel in your tech career.
Learn from basic to advance coding skills and become a better programmer:
- Datastructure and Algorithms
- System Design
- Programming Languages like Java, Python, and more
And Much More: Stay tuned for a variety of content that will take your coding skills to the next level.
Whether you're prepping for an upcoming interview or looking to broaden your programming knowledge, Code with K5KC is here to support you every step of the way. Subscribe and join our community of learners and future tech leaders! 🚀
Max Subarray | LeetCode 53 | 4 Methods: Naive, Divide & Conquer, Kadane's Algorithm
Problem Description
Given an integer array nums, find the contiguous subarray (containing at least one number) which has the largest sum and return its sum.
Problem: leetcode.com/problems/maximum-subarray/
Code link: k5kc.com/cs/algorithms/maximum-subarray-sum
Given an integer array nums, find the contiguous subarray (containing at least one number) which has the largest sum and return its sum.
Problem: leetcode.com/problems/maximum-subarray/
Code link: k5kc.com/cs/algorithms/maximum-subarray-sum
Просмотров: 18
Видео
Target Sum - Leetcode 494 - Java
Просмотров 194 часа назад
Problem Description You are given an integer array nums and an integer target. You want to build an expression out of nums by adding one of the symbols ' ' and '-' before each integer in nums and then concatenate all the integers. For example, if nums = [2, 1], you can add a ' ' before 2 and a '-' before 1 and concatenate them to build the expression " 2-1". Return the number of different expre...
Is Subsequence - Leetcode 392
Просмотров 89 часов назад
Problem Description Given two strings s and t, return true if s is a subsequence of t, or false otherwise. OR Given two strings str1 and str2, find if str1 is a subsequence of str2. Problem: leetcode.com/problems/is-subsequence/ Code link: k5kc.com/cs/algorithms/is-subsequence-problem/
Minimum Number of Operations to Sort a Binary Tree by Level - Leetcode 515 - Java
Просмотров 199 часов назад
Problem Description Given the root of a binary tree, return an array of the largest value in each row of the tree (0-indexed). Problem: leetcode.com/problems/find-largest-value-in-each-tree-row/ Code link: k5kc.com/cs/algorithms/find-largest-value-in-each-tree-row-problem/
Minimum Number of Operations to Sort a Binary Tree by Level - Leetcode 2471 - Java
Просмотров 2212 часов назад
Problem Description You are given the root of a binary tree with unique values. In one operation, you can choose any two nodes at the same level and swap their values. Return the minimum number of operations needed to make the values at each level sorted in astrictly increasing order. The level of a node is the number of edges along the path between it and the root node. Problem: leetcode.com/p...
Binary Tree Level Order Traversal - Leetcode 102 - Java
Просмотров 2312 часов назад
Problem Description Given the root of a binary tree, return the level order traversal of its nodes' values. (i.e., from left to right, level by level). Problem: leetcode.com/problems/binary-tree-level-order-traversal/ Code link: k5kc.com/cs/algorithms/binary-tree-level-order-traversal-level-by-level/
Level Order Traversal - Detailed Explanation
Просмотров 4414 часов назад
Learn how to perform a level-order traversal of a binary tree in Java! This video walks through the code step-by-step and explains the logic behind using a queue for efficient traversal. Code link: k5kc.com/cs/algorithms/binary-tree-level-order-traversal/
Reverse Odd Levels of Binary Tree - Leetcode 2415 - Java
Просмотров 1121 час назад
Problem Description Given the root of a perfect binary tree, reverse the node values at each odd level of the tree. For example, suppose the node values at level 3 are [2,1,3,4,7,11,29,18], then it should become [18,29,11,7,4,3,1,2]. Return the root of the reversed tree. A binary tree is perfect if all parent nodes have two children and all leaves are on the same level. The level of a node is t...
Max Chunks to Make Sorted - Leetcode 769 - Java
Просмотров 16День назад
Problem Description You are given an integer array arr of length n that represents a permutation of the integers in the range [0, n - 1]. We split arr into some number of chunks (i.e., partitions), and individually sort each chunk. After concatenating them, the result should equal the sorted array. Return the largest number of chunks we can make to sort the array. Problem: leetcode.com/problems...
Final Prices With a Special Discount in a Shop - Leetcode 1475 - Java
Просмотров 12День назад
Problem Description You are given an integer array prices where prices[i] is the price of the ith item in a shop. There is a special discount for items in the shop. If you buy the ith item, then you will receive a discount equivalent to prices[j] where j is the minimum index such that j > i and prices[j] <= prices[i]. Otherwise, you will not receive any discount at all. Return an integer array ...
Construct String With Repeat Limit
Просмотров 13День назад
Problem Description You are given a string s and an integer repeatLimit. Construct a new string repeatLimitedString using the characters of s such that no letter appears more than repeatLimit times in a row. You do not have to use all characters from s. Return thelexicographically largest repeatLimitedString possible. A string a is lexicographically larger than a string b if in the first positi...
Final Array State After K Multiplication Operations I - Leetcode 3264 - Java
Просмотров 13День назад
Problem Description You are given an integer array nums, an integer k, and an integer multiplier. You need to perform k operations on nums. In each operation: Find the minimum value x in nums. If there are multiple occurrences of the minimum value, select the one that appears first. Replace the selected minimum value x with x * multiplier. Return an integer array denoting the final state of num...
Find Score of an Array After Marking All Elements - Leetcode 2593 - Java
Просмотров 4114 дней назад
Problem Description You are given an array nums consisting of positive integers. Starting with score = 0, apply the following algorithm: - Choose the smallest integer of the array that is not marked. If there is a tie, choose the one with the smallest index. - Add the value of the chosen integer to `score`. - Mark the chosen element and its two adjacent elements if they exist . - Repeat until a...
Take Gifts From the Richest Pile
Просмотров 1414 дней назад
Problem Description You are given an integer array gifts denoting the number of gifts in various piles. Every second, you do the following: Choose the pile with the maximum number of gifts. If there is more than one pile with the maximum number of gifts, choose any. Leave behind the floor of the square root of the number of gifts in the pile. Take the rest of the gifts. Return the number of gif...
Special Array II - Leetcode 3152 - Java
Просмотров 6414 дней назад
Problem Description An array is considered special if every pair of its adjacent elements contains two numbers with different parity. You are given an array of integer nums and a 2D integer matrix queries, where for queries[i] = [fromi, toi] your task is to check that subarray nums[fromi..toi] is special or not. Return an array of booleans answer such that answer[i] is true if nums[fromi..toi] ...
Maximum Number of Integers to Choose From a Range I - Leetcode 2554 - Java
Просмотров 2921 день назад
Maximum Number of Integers to Choose From a Range I - Leetcode 2554 - Java
Move Pieces to Obtain a String - Leetcode 2337 - Java
Просмотров 3521 день назад
Move Pieces to Obtain a String - Leetcode 2337 - Java
Make String a Subsequence Using Cyclic Increments - Leetcode 2825 - Java
Просмотров 2621 день назад
Make String a Subsequence Using Cyclic Increments - Leetcode 2825 - Java
Maximum XOR for Each Query - Leetcode 1829 - Java
Просмотров 25Месяц назад
Maximum XOR for Each Query - Leetcode 1829 - Java
Largest Combination With Bitwise AND Greater Than Zero - Leetcode 2275 - Java
Просмотров 39Месяц назад
Largest Combination With Bitwise AND Greater Than Zero - Leetcode 2275 - Java
Find if Array Can Be Sorted - Leetcode 3011 - Java | 3 Approaches
Просмотров 68Месяц назад
Find if Array Can Be Sorted - Leetcode 3011 - Java | 3 Approaches
Minimum Number of Changes to Make Binary String Beautiful - Leetcode 2914 - Java
Просмотров 84Месяц назад
Minimum Number of Changes to Make Binary String Beautiful - Leetcode 2914 - Java
String Compression III - Leetcode 3163 - Java
Просмотров 101Месяц назад
String Compression III - Leetcode 3163 - Java
Circular Sentence - Leetcode 2490 - Java
Просмотров 34Месяц назад
Circular Sentence - Leetcode 2490 - Java
Delete Characters to Make Fancy String - Leetcode 1957 - Java
Просмотров 69Месяц назад
Delete Characters to Make Fancy String - Leetcode 1957 - Java
Remove Sub-Folders from the Filesystem - Leetcode 1233 - Java
Просмотров 282 месяца назад
Remove Sub-Folders from the Filesystem - Leetcode 1233 - Java
Longest Square Streak in an Array - Leetcode 2501 - Java
Просмотров 312 месяца назад
Longest Square Streak in an Array - Leetcode 2501 - Java
Height of Binary Tree After Subtree Removal Queries - Leetcode 2458 - Java
Просмотров 372 месяца назад
Height of Binary Tree After Subtree Removal Queries - Leetcode 2458 - Java
Flip Equivalent Binary Trees - Leetcode 951 - Java
Просмотров 482 месяца назад
Flip Equivalent Binary Trees - Leetcode 951 - Java
this was asked in my interview OA, i calculated the cost before hand by storing the cost of operation for each index into an array and then just used it while checking for the answer
Nice, that appraoch should work as well, and seems efficient to me. But there is a trade-off I guess, and that is with slightly higher space complexity. But most important thing is you solved that problem during the interview, and that is what matters!
excellent explanation thank you
Thanks
Excellent explanation, thank you
great
❤❤❤❤
Happy Coding 😊
Thank you 🫰
Very well explained!
NICE 🎉
Nice explanation
I saw this solution in TS, it was so confusing but this explanation cleared everything up. It makes a lot of sense, it's like a sliding window, you're just upping the frequency of each number already present when shifting each duplicate to become a unique number wow. Ty!
Glad that it makes sense to you :)
Nice Video, straight to the point and the slides are well made