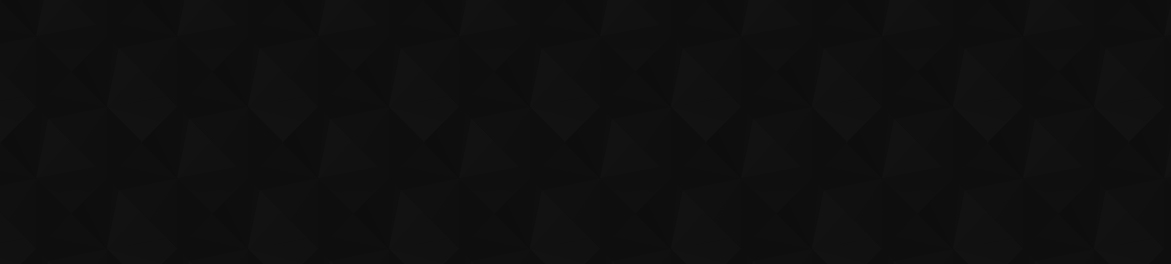
- Видео 398
- Просмотров 232 413
CodeByHeart
Индия
Добавлен 14 июн 2021
A long journey of skill development
#FreeEducation
#freeeducationforall
I am training highly interested people in JavaScript, NodeJS, TypeScript, MongoDB, MySQL, AWS, and other tech stacks. It is 100% practical, hands-on training; you will learn by doing.
The purpose is to provide quality and prepare a team of individuals who can start working independently & do end-to-end work by themselves. crack interviews with confidence, and perform excellently in their workspace.
To get a customized learning road map(Focus on MERN stack) for you, write an email to sayedazharsabri@gmail.com and in the subject write "Career Guidance: Your_Name"
#FreeEducation
#freeeducationforall
I am training highly interested people in JavaScript, NodeJS, TypeScript, MongoDB, MySQL, AWS, and other tech stacks. It is 100% practical, hands-on training; you will learn by doing.
The purpose is to provide quality and prepare a team of individuals who can start working independently & do end-to-end work by themselves. crack interviews with confidence, and perform excellently in their workspace.
To get a customized learning road map(Focus on MERN stack) for you, write an email to sayedazharsabri@gmail.com and in the subject write "Career Guidance: Your_Name"
Master Function Memoization in JavaScript | Boost Performance with Code Optimization
Write a program to create memoized version of that function
Discover how to harness the power of memoization in JavaScript to enhance your function performance. Memoization is a game-changing technique that can significantly speed up your code and improve efficiency.
Key questions we'll address:
- How to memoize a function?
- What's the practical implementation of memoization?
- How does memoization enhance performance?
- What's the internal workings of a memoized function?
- Can memoization be applied in React applications?
This tutorial covers:
- Core concepts of memoization
- Converting simple functions into memoized versions
- Implementing memoization with closures
- Edge cases and limitations of...
Discover how to harness the power of memoization in JavaScript to enhance your function performance. Memoization is a game-changing technique that can significantly speed up your code and improve efficiency.
Key questions we'll address:
- How to memoize a function?
- What's the practical implementation of memoization?
- How does memoization enhance performance?
- What's the internal workings of a memoized function?
- Can memoization be applied in React applications?
This tutorial covers:
- Core concepts of memoization
- Converting simple functions into memoized versions
- Implementing memoization with closures
- Edge cases and limitations of...
Просмотров: 23
Видео
Restrict a function to be called only once | Interview | Important
Просмотров 32Месяц назад
In this video, we'll explore a powerful technique in JavaScript: creating a function that ensures another function is called at most once. This concept can be useful in scenarios where you need to prevent a function from being executed multiple times, such as initializing a component or setting up event listeners. Here's what we'll cover: 🔹 Understanding the importance of controlling function e...
Write a program to return length of Arguments Passed to the function
Просмотров 122Месяц назад
In this video, we'll explore a simple yet useful technique in JavaScript: returning the length of arguments passed to a function. This concept can be helpful in various scenarios, such as validating the number of arguments, handling optional parameters, or creating flexible functions that can work with varying numbers of arguments. Here's what we'll cover: 🔹 Understanding the importance of argu...
Creating a New Function from an Array of Functions | Interview | Important
Просмотров 84Месяц назад
Mastering Function Composition in JavaScript: Creating a New Function from an Array of Functions GitHub:- github.com/sayedazharsabri/workshop-content/blob/main/nodeJS-interview-questions/README.md In this video, we delve into the concept of function composition and learn how to create a new function that is the composition of an array of functions in JavaScript. This powerful technique allows y...
How to create your own reduce function for integer array | Interview | Important | JS [Hindi/Urdu]
Просмотров 28Месяц назад
How to create your own reduce function for integer array GitHub:- github.com/sayedazharsabri/workshop-content/blob/main/nodeJS-interview-questions/README.md In this video, we'll explore the concept of array reduction and learn how to create our own reduce function in JavaScript. We'll solve the problem of reducing an integer array based on a given reducer function and an initial value, without ...
How to create your own filter function for integer array
Просмотров 552 месяца назад
Custom filter function for integer array GitHub:- github.com/sayedazharsabri/workshop-content/blob/main/nodeJS-interview-questions/README.md In this video, we'll explore the concept of array filtering and learn how to create our own filter function in JavaScript. We'll solve the problem of filtering an integer array based on a given condition without using the built-in Array.filter method. Here...
How to create your own map function for integer array | Interview | Array
Просмотров 282 месяца назад
How to create your own map function for integer array GitHub:- github.com/sayedazharsabri/workshop-content/blob/main/nodeJS-interview-questions/README.md Apply Transform Over Each Element in Array Given an integer array arr and a mapping function fn, return a new array with a transformation applied to each element. The returned array should be created such that returnedArray[i] = fn(arr[i], i)....
How to Reset WSL Ubuntu Password on Windows A Step by Step Guide [Hindi/Urdu]
Просмотров 3242 месяца назад
Reset WSL Ubuntu Password on Windows: A Step-by-Step Guide The Windows Subsystem for Linux (WSL) allows you to run a Linux distribution, such as Ubuntu, directly on your Windows machine. However, there may be times when you need to reset your WSL Ubuntu password, either for the root user or your regular user account. In this video, we'll provide a comprehensive guide on how to reset the WSL Ubu...
Write a counter function with increment decrement and reset options
Просмотров 432 месяца назад
GitHub:- github.com/sayedazharsabri/workshop-content/blob/main/nodeJS-interview-questions/README.md closure: ruclips.net/video/imE9YgHi194/видео.html In this video, we'll explore the powerful concept of closures in JavaScript by creating a versatile createCounter function. This function allows you to create a counter object with three methods: increment, decrement, and reset. Here's what we'll ...
Write a function to check To Be Or Not To Be | Build Your Own 'expect' Function | Interview
Просмотров 432 месяца назад
Simplified Unit Testing with JavaScript: Build Your Own 'expect' Function GitHub:- github.com/sayedazharsabri/workshop-content/blob/main/nodeJS-interview-questions/README.md closure: ruclips.net/video/imE9YgHi194/видео.html Unit testing is an essential practice in software development, helping you catch bugs early, maintain code quality, and ensure your application's functionality. While there ...
Write a counter function using closure and provide option to set the starting value | Interview
Просмотров 382 месяца назад
Mastering Closures in JavaScript: Build a Flexible Counter Function Write a counter function using closure and provide option to set the starting value GitHub:- github.com/sayedazharsabri/workshop-content/blob/main/nodeJS-interview-questions/README.md Learn closure: ruclips.net/video/Cfl-e2h60fA/видео.html Closures are a powerful concept in JavaScript that allow functions to access and "remembe...
Write a function and It should return a new function that always returns Hello World
Просмотров 373 месяца назад
Write a function createHelloWorld. It should return a new function that always returns "Hello World" GitHub: github.com/sayedazharsabri/workshop-content/blob/main/nodeJS-interview-questions/README.md In this video, we'll explore the concept of higher-order functions in JavaScript by creating a simple yet powerful function called createHelloWorld. Here's what we'll cover: 🔹 Understanding higher-...
What is a Callback and How to Handle Callback Hell in JavaScript
Просмотров 1123 месяца назад
What is a Callback and How to Handle Callback Hell in JavaScript GitHub:- github.com/sayedazharsabri/workshop-content/blob/main/nodeJS-interview-questions/README.md Callbacks are a fundamental concept in JavaScript, essential for handling asynchronous operations and enabling non-blocking, event-driven programming. However, as your codebase grows and becomes more complex, you may find yourself t...
Effortlessly Duplicate Arrays in JavaScript with the Duplicator Method | Interview | Important
Просмотров 553 месяца назад
Effortlessly Duplicate Arrays in JavaScript with the Duplicator Method GitHub:- github.com/sayedazharsabri/workshop-content/blob/main/nodeJS-interview-questions/README.md In this video, we'll explore a powerful technique to duplicate arrays in JavaScript using a custom Duplicator method. This handy method will allow you to create a new array that contains multiple copies of the original array's...
Find the Biggest Number with Math.max() in JavaScript | Important Interview Que
Просмотров 864 месяца назад
Find the Biggest Number with Math.max() in JavaScript | Important Interview Que
How to set prefix before every line you log | Important interview question
Просмотров 344 месяца назад
How to set prefix before every line you log | Important interview question
Exception Handling in NodeJS | Important Interview Question
Просмотров 1354 месяца назад
Exception Handling in NodeJS | Important Interview Question
Master Date Manipulation in JavaScript: Add Custom Methods for Getting Next Date
Просмотров 474 месяца назад
Master Date Manipulation in JavaScript: Add Custom Methods for Getting Next Date
How to serve static files and images from NodeJS Express server
Просмотров 1596 месяцев назад
How to serve static files and images from NodeJS Express server
How do you check that 2 is passed to a function or not | Practical Interview | validation function
Просмотров 966 месяцев назад
How do you check that 2 is passed to a function or not | Practical Interview | validation function
How to pass an arbitrary object as this | bind | call | Practical interview question
Просмотров 647 месяцев назад
How to pass an arbitrary object as this | bind | call | Practical interview question
Create Array.prototype.last() | JavaScript Practical | Interview
Просмотров 1717 месяцев назад
Create Array.prototype.last() | JavaScript Practical | Interview
What and Why Spaghetti Code in JavaScript and Prevention | Interview | Important [Hindi/Urdu]
Просмотров 1127 месяцев назад
What and Why Spaghetti Code in JavaScript and Prevention | Interview | Important [Hindi/Urdu]
Revealing Module Pattern | JavaScript Design Pattern | Interview question [Hindi]
Просмотров 2497 месяцев назад
Revealing Module Pattern | JavaScript Design Pattern | Interview question [Hindi]
The Nullish Coalescing Operator (??) | ES2020 [Hindi]
Просмотров 877 месяцев назад
The Nullish Coalescing Operator (??) | ES2020 [Hindi]
PostgreSQL Installation on Windows 11 | Step-by-Step Guide [English]
Просмотров 22310 месяцев назад
PostgreSQL Installation on Windows 11 | Step-by-Step Guide [English]
Introduction to Database | SQL vs NoSQL | Why and when to choose which database
Просмотров 25311 месяцев назад
Introduction to Database | SQL vs NoSQL | Why and when to choose which database
Shallow Copy vs Deep Copy | Reference vs Copy | Library & Program for Deep Copy | Array | Object
Просмотров 17911 месяцев назад
Shallow Copy vs Deep Copy | Reference vs Copy | Library & Program for Deep Copy | Array | Object
thanks
Always welcome
I installed in windows It's working properly but When i check the terraform --version It's showing command not found I already installed in my window system. Help mee brooo..
If you are facing the problem with installed software inside WSL. Possibly the issue is with the persistency of the PATH. Simply search it to persist the PATH inside WSL on windows and you will get answer
Thank you so much❤
You're welcome 😊
best explanation in youtube easy and readable
thanks a lot
Thanku Very Much I was found my problem solution with your helpful video
Glad to hear that
bhai yar help krdo plz
Kya diqqat a rahi hai?
@@codebyheart how can we make multiple user accounts like parallel space present in mobile phones by default , in windows private users like function in phone , i want to use a different fingerprint for every user that only i could know and nobody can find other user accounts that how many users are in laptop , sometimes we can see user profiles in settings but i want to hide every account even in boot or login page like it should be a secret window laptop irrespective of other user data or even folder , or apps or anything , i want to make private window data for me , and other user accounts some for work , office , management , one for linux etc . that doesn't even should show that other user have made a folder or installed an app it shouldn't be seen in other user accounts, like in one laptop 2 or 3 different laptop (user) working privately that nobody can't find only i should know . i think i have explained to u correctly ? please give me some solution. I can't find videos related to this . or i don't know how to search .
@@codebyheart how can we make multiple user accounts like parallel space present in mobile phones by default , in windows private users like function in phone , i want to use a different fingerprint for every user that only i could know and nobody can find other user accounts that how many users are in laptop , sometimes we can see user profiles in settings but i want to hide every account even in boot or login page like it should be a secret window laptop irrespective of other user data or even folder , or apps or anything , i want to make private window data for me , and other user accounts some for work , office , management , one for linux etc . that doesn't even should show that other user have made a folder or installed an app it shouldn't be seen in other user accounts, like in one laptop 2 or 3 different laptop (user) working privately that nobody can't find only i should know . i think i have explained to u correctly ? please give me some solution. I can't find videos related to this . or i don't know how to search .
@@codebyheart how can we make multiple user accounts like parallel space present in mobile phones by default , in windows private users like function in phone , i want to use a different fingerprint for every user that only i could know and nobody can find other user accounts that how many users are in laptop , sometimes we can see user profiles in settings but i want to hide every account even in boot or login page like it should be a secret window laptop irrespective of other user data or even folder , or apps or anything , i want to make private window data for me , and other user accounts some for work , office , management , one for linux etc . that doesn't even should show that other user have made a folder or installed an app it shouldn't be seen in other user accounts, like in one laptop 2 or 3 different laptop (user) working privately that nobody can't find only i should know . i think i have explained to u correctly ? please give me some solution. I can't find videos related to this . or i don't know how to search .
Thank you so much sir for this react series
Always welcome
Useful concept. It would be even more helpful if you also explained how to solve this problem using recursion, in addition to the iterative approach.
Thanks, yes, that is what I am doing in programming classes, In this series, I want to explain everything separately.
thanks
You're welcome!
thank you it worked
Glad it helped
Thank you so much❤❤❤
Always welcome
Thank you Sir ❤
Always welcome
Thanks bro
Welcome brother
Awesome explain !!
Glad you liked it!
Thank you so much.. i had watched every video on youtube but couldn’t help me and after two days this video has helped me.
Glad it helped, always welcome
thanks sir ❤❤
Most welcome
Sir ESLINT Failed to load config "react-app" to extend from error aa raha hai solution do please ❤
Please share the complete error and also the version of Node, React, and Eslint.
Nice explanation
Thanks dear
As we are moving forward in this series its become difficult to understand.
Practice makes the things easier, implement it while watching the video and then implement it after watching the video and create some short notes, these all concepts are really important to understand. All the very best
Dhanyawaad Guru....❤
Swagatam
It is very easy to understand the concepts with your videos sir.
Glad to hear that
Thanks bro
Always Welcome
I have done this login functionality I'll practice to grab each concept
All the best
Thank you so much sir
Most welcome
Great explanation! Really helped me understand how to work with routers and controllers in Node.js. Thanks for the clear demonstration! ❤
You are welcome!
hi sir wsl kasa use karo window 10 maplz plz bata da
Mere paas windows 10 nahi hai ab, so video nahi bana sakta hun. microsoft ka page hai ise ek baar dekhiye - learn.microsoft.com/en-us/windows/wsl/install
not working getting 403 ☹☹, though i am able to open file using link in browser but not from react or simple html page, is something updated?
As I know nothing updated, when you are opening from browser that is different, and when using it from application then its different. Is drive directory is public ?
@@codebyheart yes it is public and got it working with drive.google.com/thumbnail?id= this link, but the mentioned in the video not working for me 🫤
👍
Thanks
👍👍👍👍
Thanks
nice👍👍👍👍👍👍
Thanks
👍👍👍👍👍👍👍👍
Thanks
Finally I have done it
Great, All the best
Best javascript tutorial on youtube! I like this most 👍❤
Glad you think so!
❤
Thanks a lot
Thanks bro
Always welcome brother
Thank you sir
Always Welcome Dear
Your explanations are so clear and concise! Thank you for making complex concepts easy to understand.👍
Glad you think so!
You've made me excited to learn more.👍
Awesome! Thank you!
Your videos are always well-structured and engaging. You're an amazing teacher!
Glad you like them!
Thank you for sharing your knowledge and expertise with the world. You're making a real difference,you are the best mentor👍
So nice of you
I'm so grateful for your dedication and your willingness to help others learn. You're the best!👍
Thank you so much
You're an inspiration to many, and your teaching style is exceptional. Keep it up, Thankyou
Thanks, will do!
Bhai error aa raha he 0×80370102
Please write the full error and also the configuration of windows
👍👍
Thanks
👍
Thanks
Thanks a lot for sharing these valuable lectures for us , I am very happy to find a teacher like u, your lectures are best gift for me👍
It's my pleasure, thanks a lot
Always provide excellent content.Thank you so much.
So nice of you, Always welcome
👍lot of thanks for this valuable content👍
My pleasure
Now concept of closure is clear , thankyou 👍
Glad to hear that
Great👍
Thanks